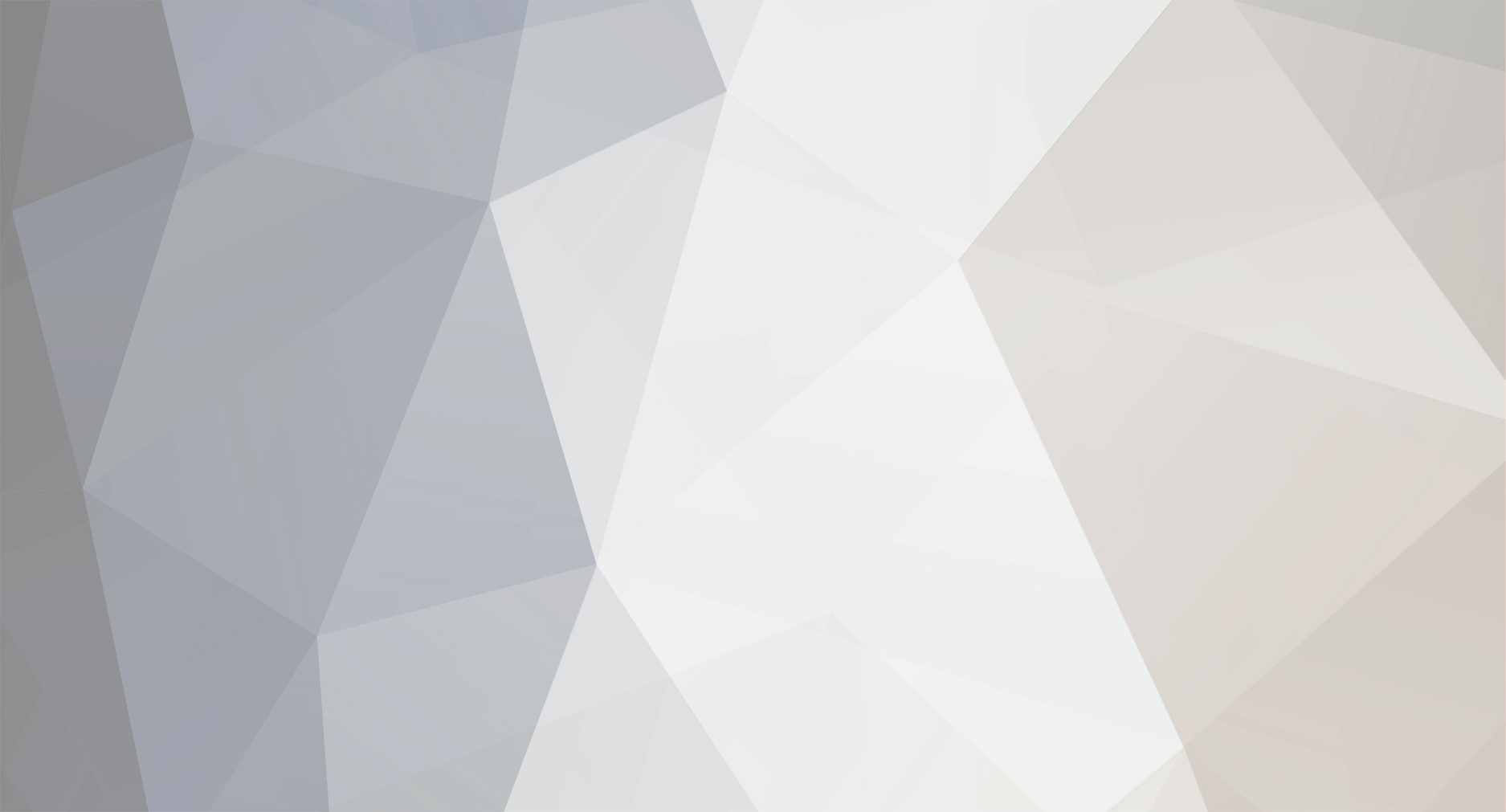
BeeDev
Member-
Posts
328 -
Joined
-
Last visited
-
Days Won
2
Content Type
Profiles
Forums
Events
Downloads
Gallery
Store
Everything posted by BeeDev
-
- ID should be generated automatically by the database's "autonumber" field. You shouldn't need a counter for that. You only need ID's when updating a row. - Don't forget to attach a random string as a querystring to your AJAX url so the browser doesn't cache the result, and returns "Success" everytime even if there was an error. Also good practice to "Disable" any buttons during the AJAX submission to prevent any double-entries. - Think it would be nice to update the current table with newly added records once they've been inputted. Maybe another AJAX page that returns all records as "<table>" in html format, or just an array/json can do the job as well.
-
You're welcome. I guess a small correction would be to move e.preventDefault() outside the "if statement" as it should run everytime regardless if the link is the active one or not. // Get all anchors with ID starting with nav var $nav = $("a[id^=nav]"); // Get all h3 with ID starting with section var $sec = $("h3[id^=section]"); // Declare $this var $this; $nav.each(function(i){ $this = $(this); $this.bind("click",function(e){ // cancel default anchor click event behavior ... // ... same as "return false" but doesn't stop the further execution of code e.preventDefault(); if(!$this.hasClass("active")){ // clear all currently active subnav items & activate current subnav item $nav.removeClass("active"); $this.addClass("active"); // hide current active section and remove active class $("h3.active").removeClass("active").next("div.show").animate({ opacity : 'toggle', height: 'toggle' }, 'slow'); // show the section that was clicked on $sec.eq(i).addClass("active").next("div.show").animate({ opacity : 'toggle', height: 'toggle' }, 'slow'); } }); }); There's also a way to avoid the weird bouncing effect that results from resize 2 divs at the same time. It's a little bit complicated to explain, but basically the jQuery.animate() function has few callbacks, and one of them is called "step". You can basically assign a function to this which gets fired on every step of the animation. This way you make sure that when div.a reduces by 2px div.b increases by 2px. But use .css() instead of .animate() inside the step() callback. Anyway probably this level of careful animation is not needed
-
// Get all anchors with ID starting with nav var $nav = $("a[id^=nav]"); // Get all h3 with ID starting with section var $sec = $("h3[id^=section]"); $nav.each(function(i){ var $this = $(this); $this.bind("click",function(e){ if(!$this.hasClass("active")){ // cancel default anchor click event behavior ... // ... same as "return false" but doesn't stop the further execution of code e.preventDefault(); // clear all currently active subnav items & activate current subnav item $nav.removeClass("active"); $this.addClass("active"); // hide current active section and remove active class $("h3.active").removeClass("active").next("div.show").animate({ opacity : 'toggle', height: 'toggle' }, 'slow'); // show the section that was clicked on $sec.eq(i).addClass("active").next("div.show").animate({ opacity : 'toggle', height: 'toggle' }, 'slow'); } }); }); notice the variable 'i' being passed to the each function. This has the counter starts from 0. And this counter is being used in the last part to reference the correct #sectionX item: // show the section that was clicked on $sec.eq(i).next("div.show").animate({ opacity : 'toggle', height: 'toggle' }, 'slow').addClass("active"); .eq() function's name is an abbreviation of "equals" i'm guessing here. Basically if you pass it a number, it will select the n'th item from the object array. $sec.eq(i) will select the 2nd item if i=1, and i=1 will only be true if the 2nd link (#nav) is clicked on. I haven't tested this code but it should work, if not I trust you can debug it yourself And why use a 15kb Javascript library when you can achieve the same result with 1kb? var $nav=$("a[id^=nav]");var $sec=$("h3[id^=section]");$nav.each(function(i){var $this=$(this);$this.bind("click",function(e){if(!$this.hasClass("active")){e.preventDefault();$nav.removeClass("active");$this.addClass("active");$("h3.active").next("div.show").animate({opacity:'toggle',height:'toggle'},'slow').removeClass("active");$sec.eq(i).next("div.show").animate({opacity:'toggle',height:'toggle'},'slow').addClass("active");}});}); (minified with http://www.ventio.se/tools/minify-js/ )
-
I'd suggest using <table>
-
Very similar to YSlow but from Google (Installs as Chrome or Firefox/Firebug plugin): http://code.google.com/speed/page-speed/ Also I use this at the end of projects to minify my CSS document (It removes all the white space/line breaks/comments etc from your CSS and returns a CSS that's only 1 line): http://www.ventio.se/tools/minify-css/ Note: Please make a backup of your original file in case you need to edit it again Also for optimizing images this site is good: http://www.smushit.com/ysmush.it/ It's made by Yahoo, all you need to do is provide a URL or upload an image file and it will return an optimised one for you to download. Sometimes cuts image sizes in half! Sometimes only by a little bit.
-
Haven't managed to go through your code, but I just wanted to suggest a few things in terms of the questions you asked. Firstly, how to display files for download when you've changed the names. This one is quite easy actually, you just need to store 2 file names for each file, first is the md5 name which will be the actual filename, and second will be the "old" filename (mysecuredoc.doc for example) and this will only be used to display the links. In terms of actually securely downloading, I have set this up before. But I'm not sure how secure it is ... Basically the idea is to have a download page, which checks the session if the person requesting the download has the right login credentials etc, then after the checks pass, the download page gets the md5 file name from the database (a querystring with the display name, or ID of database record probably needs to be passed to this page). However instead of forwarding the user to that file, you need to serve the file from the download page. To do that it's quite simple you just set some headers, and then fopen (php function) the file and send it. For this to work, you will need to store the filetype (MIME type) in the database as well, to create a correct header. Sample code below which i've written long ago for a site, but it's just to give you and idea. $f contains the file path and name (for example: "/home/mysite.com/secure/files/23123kjlk3231.doc"), and $af contains the display name (for example: "mysecuredoc.doc") which needs to be URL Encoded, and last parameter $ftype is the file MIME type. function sendFile($f, $af, $ftype) { // common MIME types: // use application/msword for .doc files // use application/vnd.openxmlformats-officedocument.wordprocessingml.document for .docx // use application/octet-stream for any binary file // use application/x-executable-file for executables // use application/x-zip-compressed for zip files // use application/pdf for pdf documents // Visit http://filext.com/faq/office_mime_types.php for more MS Office Mime Types if($f != '') { header("Content-Type: ".$ftype); header("Content-Length: " . filesize($f)); header("Content-Disposition: attachment; filename=\"$af\""); header("Cache-Control: must-revalidate, post-check=0, pre-check=0"); $fp = fopen($f,"r"); fpassthru($fp); fclose($fp); return true; }else{ return; } } Hope this is useful. Good luck
-
If it's just to keep the right column separate then you can use normal <div> elements. But make the <div> of your right column have "position:fixed" css attribute. Then it will stick on the side of the page even though you scroll the main body. Easiest example would be the bottom panel on facebook which sticks to the bottom of the browser as you scroll, which effectively keeps it "separate". If you want more info then just google "position fixed" and you will get some articles, and also how to trick Internet Explorer 6 (because it doesn't know what position:fixed is )
-
it's called a Fade effect. These effects can be very easily achieved using the recent Javascript libraries such as: jQuery, mooTools, Scriptaculous and YUI Library etc. Basically it's manipulating the element's opacity incrementally using a loop probably, so it appears as though it's fading in and fading out.
-
It's good practice to use semi-colon ( ; ) at end of each line in Javascript, also to use opening and closing brackets on if statements etc. Also it's good practice to use ID's when you're doing these things, instead of name, so you can more accurately target elements, and also it works consistently across different browsers. In your case, put an ID on the image: <img id="slideshow" src="images/qdimages/eth.jpg" border=0 width="242px" height="190px"> Notice the id "slideshow" above Also you should maybe use an "onload" event to fire the slideshowimages() and slideit() functions: <script> window.onload = function(){ slideshowimages("images/qdimages/eth1.jpg","images/qdimages/eth2.jpg","images/qdimages/jonica1.jpg","images/qdimages/jonica3.jpg","images/qdimages/jonica4.jpg","images/qdimages/jonica5.jpg"); slideit(); }; </script> Here's the code that I've tested just now and confirm it's working on IE6 and FF3.6: var slideimages = new Array(); var slideshowspeed = 4000; var whichimage=0; var t; function slideshowimages(){ for (i=0;i<slideshowimages.arguments.length;i++){ slideimages[i] = new Image(); slideimages[i].src = slideshowimages.arguments[i]; } } function slideit(){ if(!document.getElementById("slideshow")){ return; } document.getElementById("slideshow").src = slideimages[whichimage].src; if(whichimage<slideimages.length-1){ whichimage++; }else{ whichimage=0; } t = setTimeout(slideit, slideshowspeed); }
-
A sound knowledge of CSS and Javascript is probably required to code with jQuery. But don't let that steer you away from it, from my experience jQuery has the lowest learning curve out of all the other Javascript libraries such as YUI, mooTools, Scriptaculous etc. A good place to start is the jQuery website. Try to go through their Tutorials, and also Documentation (start with Getting Started). If you don't understand anything that's there, then it might be a good idea to go for your Javascript book or Stefan's videos
-
$dbLink is taking a value inside an "if" statement. So if that IF statement is not true, $dbLink will never get a value, thus it will be "undeclared". So when that "if" statement fails, $dbLink has no value, but going by the program logic it will still try to execute the line $dbLink->close(), but it will fail miserably because $dbLink doesn't have any value, so trying to close() it will not work and so it gives you the error So close the SQL Connection in the same context (context = the same IF statement that it was declared & opened in). See below: <?php // Check if a file has been uploaded if(isset($_FILES['uploaded_file'])) { // Make sure the file was sent without errors if($_FILES['uploaded_file']['error'] == 0) { // Connect to the database $dbLink = new mysqli('localhost', 'root', '', 'blob'); if(mysqli_connect_errno()) { die("MySQL connection failed: ". mysqli_connect_error()); } // Gather all required data $name = $dbLink->real_escape_string($_FILES['uploaded_file']['name']); $mime = $dbLink->real_escape_string($_FILES['uploaded_file']['type']); $data = $dbLink->real_escape_string(file_get_contents($_FILES ['uploaded_file']['tmp_name'])); $size = intval($_FILES['uploaded_file']['size']); // Create the SQL query $query = " INSERT INTO `testblob` ( `name`, `mime`, `size`, `data`, `created` ) VALUES ( '{$name}', '{$mime}', {$size}, '{$data}', NOW() )"; // Execute the query $result = $dbLink->query($query); // Check if it was successfull if($result) { echo 'Success! Your file was successfully added!'; } else { echo 'Error! Failed to insert the file' . "<pre>{$dbLink->error}</pre>"; } //Close the mysql connection $dbLink->close(); } else { echo 'An error accured while the file was being uploaded. ' . 'Error code: '. intval($_FILES['uploaded_file']['error']); } } else { echo 'Error! A file was not sent!'; } // Echo a link back to the main page echo '<p>Click <a href="index.html">here</a> to go back</p>'; ?>
-
Firefox to IE or IE to Firefox - compatibility problems
BeeDev replied to markwill2112's topic in CSS
When you have ONLY floated items inside a div, for example: <div class="parent"> <div class="floated_left_div"><!-- Content here --></div> <div class="another_floated_left_div"><!-- Content here --></div> </div> In the above case, the parent div doesn't stretch its height to fully contain the floated children divs. So most probably in the above case the parent div will have a height of 0px. This is a very widely known issue, and there's 2 ways to get around this problem, and have the parent div automatically adjust its height to fully contain the floated children divs. One way is as Wickham explained above: put CSS property "overflow" to value "auto" of the parent div: <div class="parent" style="overflow:auto;"> <div class="floated_left_div"><!-- Content here --></div> <div class="another_floated_left_div"><!-- Content here --></div> </div> The other way is to use "break" divs. This one is a little bit less elegant, as it involved adding unused empty tags in the markup, but nonetheless it works just as good as the above solution, and it doesn't really decrease the performance. The way to do it is put an empty div right after the floated children divs, and set its css property "clear" to value "both". For example: (Basing on above example too) <div class="parent"> <div class="floated_left_div"><!-- Content here --></div> <div class="another_floated_left_div"><!-- Content here --></div> <div style="clear:both"></div> </div> This solution only has 1 drawback that I'm aware of. Sometimes in internet explorer 6, the empty divs create an empty space, even though their height should be 0. In this case what I do is instead of putting the clear:both property on the "style" attribute of the div, i put a class="break" on the div, and in my css: div.break { clear:both; height:0px; font-size:1px; } which usually fixes the IE6 issue. Hope that explains it -
Try this: var xmlHttp = GetXmlHttpObject();; function showResult(rid, iid){ document.getElementById("iteminfo").style.display="block"; if (xmlHttp==null){ alert ("Your browser does not support features used on this page. You may need to download the latest version of Javascript. You will not be able to view the pictures and descriptions for each item."); return; } var url="pathtofilegoeshere.php"; url=url+"?rid="+rid; url=url+"&iid="+iid; xmlHttp.open("GET",url,true); xmlHttp.onreadystatechange=stateChanged; xmlHttp.send(null); } function stateChanged(){ if (xmlHttp.readyState==4 || xmlHttp.readyState=="complete"){ document.getElementById("iteminfo").innerHTML=xmlHttp.responseText; } } function GetXmlHttpObject(){ var xmlHttp=null; try{ // Firefox, Opera 8.0+, Safari xmlHttp=new XMLHttpRequest(); }catch (e){ // Internet Explorer try{ xmlHttp=new ActiveXObject("Msxml2.XMLHTTP"); }catch (e){ xmlHttp=new ActiveXObject("Microsoft.XMLHTTP"); } } return xmlHttp; } if it makes no difference then try this jQuery version: jQuery(function($){ var resultDiv = $("#iteminfo"); function showResult(rid, iid){ var url = "pathtofilegoeshere.php?rid="+rid+"&iid="&iid; $.get(url, function(data){ if(data.length > 0){ resultDiv.html(data); }else{ alert("No results returned for iid: '"+iid+"' and rid:'"+rid+"'"); } }); } });
-
I don't have a good design eye, so to me looks fine, not noticeable. Don't take my word for it tho
-
Looks ok if you zoom out of the page to imitate a large screen. But the div#stripes needs a min-width (and also possibly an IE6 min-width hack) that's equal to the #wrap width. Because when the screen width is smaller than #wrap width, 100% width of div#stripes only matches the screen size, but not the page size (Try zooming in a until scrolbars appear and scroll to the right)
-
Looks like the background image fades into a single color (or should) on the left hand side. So can you not just position the background to the right so the black area always sticks to the right hand edge of the browser, and set a background-color on the body that's same as the single color the left side is fading into? (I've checked the image and the left side color is not fading into one single color. So there has to be some editing involved to dissolve all of the left side into a single color.) If this is not viable then you can also try using an absolute positioned div that contains the background, and a separate div for the content. That way you can set the height or the width of the background div with %'s but this is really painful way of doing this. and you may need some jquery/javascript to set the heights to match Last option that I could think of is using a fixed background that's stretched across the full browser's length or height. This is not ideal for your client's requirements, but just to give you an idea, and perhaps maybe another angle that you can approach your client with. But I'm not 100% sure how this (site below) looks on a widescreen resolution. For example try the following website and try to zoom in & out. The background image stays the same, but only the text zooms in and out. http://w ww.redchat .co.uk/ Achieved by setting height 100% to html and body. Then inside body there's 2 divs, one for the content and menu, and one that contains only the background(s). The div for the background is set to 100% height and width (or maybe just height) and absolutely positioned and given z-index. The content div however has position relative (for the z-index to work on certain browsers) and z-index set bit higher than the background div. Hope this is useful, Good luck
-
Should be able to solve IE6 menu problem by setting #nav li a { display:inline } instead of block. or try float #nav li a { float: left } The right side bar with social widgets also out of position because IE6 don't support position:fixed. But there's a really easy fix for it here: http://ryanfait.com/position-fixed-ie6/
-
It's not really mentioned there, but if you want to automatically include the latest version on your pages then: <script type="text/javascript" src="http://code.jquery.com/jquery-latest.js"></script> EDIT: Or the minified version which would be more friendly for production sites: <script type="text/javascript" src="http://code.jquery.com/jquery-latest.min.js"></script>
-
Are you comparing Windows XP to the latest Mac OS ? That's not fair you know
-
Just to add, if you're using server-side languages like PHP or ASP u can do them automatically: PHP: http://localhost/test/check.php?sem=<?php echo urlencode('s1&s2'); ?>&branch=cse ASP: http://localhost/test/check.php?sem=<%=Server.URLEncode("s1&s2")%>&branch=cse
-
Ah... looks like the hasLayout bug then Add to css: #nav li a { zoom:1; } That should solve it fingers crossed.
-
/wp-content/themes/brownboutique-onecolumn/ie-styles.css This CSS has rule for #nav LI A and it has padding-top set to 4px, which is in conflict with your main stylesheet
-
Hi Eric, I have managed to fix this. This sentence gave me the solution basically, I took all the tooltips & triggers out of the div.pointer containers so all the triggers & tooltips were on same level and within a same parent - div#map. That fixed the issue beautifully. Thanks for your help. B.
-
Thanks Eric, I'll try and see if I can make it work differently.
-
Yea I have tried setting z-index for those two, and I have tried setting z-index to various other stuff but it's not working/helping. The plugin only modifies div.tooltip & sets its style to: visibility: visible; position: absolute; top: -251px; left: 35px; display: block; opacity: 0; The minus positions have something to do with the positioning of the tooltip from the relative positioned parent, instead of the viewport... not sure exactly, but had to configure the plugin in a certain way to achieve the effect of the tooltip appearing just above the mouse pointer. Before the config it was putting correct left top coordinates but it was from the view port, so all the tooltips were flowing out of the screen & creating scrollbars at the bottom of the browser. This is not a hack though, it's a simple configuration while calling the script