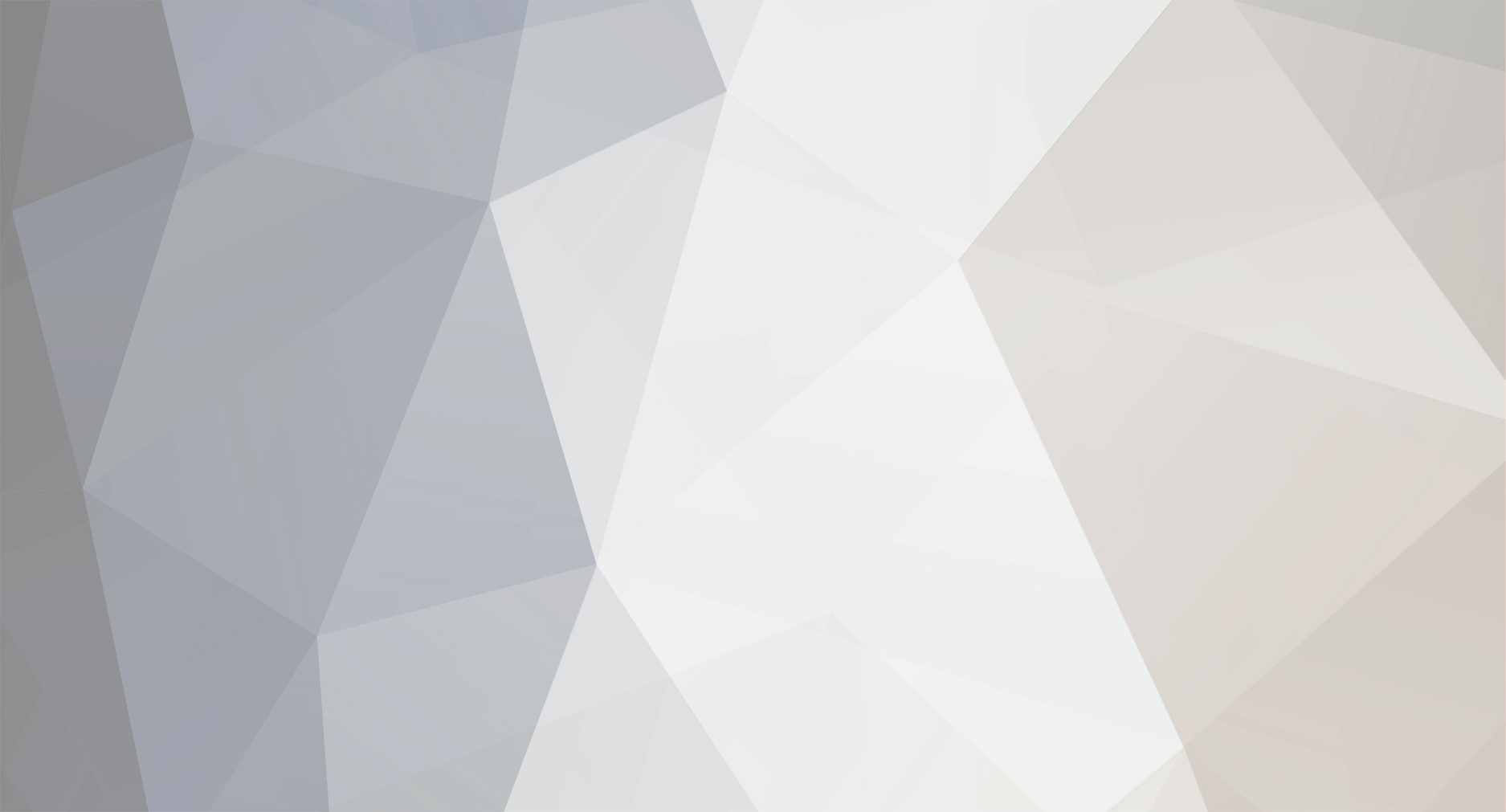
BeeDev
Member-
Posts
328 -
Joined
-
Last visited
-
Days Won
2
Content Type
Profiles
Forums
Events
Downloads
Gallery
Store
Everything posted by BeeDev
-
Just a further suggestion: there's also a property called "defaultValue" on form elements which stores the value the form field had when it first loaded. Even if you change the form field value, the defaultValue stays at the same value it was when the page loaded. So instead of comparing the values to a strings like "First name...", "Surname..." (and obviously the number of these comparisons will grow as you add more and more fields...) you can just compare it to the "defaultValue". function MM_validateForm(requiredfields) { field = requiredfields.split(","); alertmsg = ""; for(i=0; i<field.length; i++) { if(document.getElementById(field[i]) && (document.getElementById(field[i]).value=="" || document.getElementById(field[i]).value==document.getElementById(field[i]).defaultValue)) alertmsg += ", " + field[i].replace("_", ""); } if (alertmsg.length == 0) return true; else { alert("Please provide " + alertmsg.substr(1)); return false; } }
-
So from what you've written, I guess all you're asking is you can't use margin:auto to center the stuff? I just tried it and it works fine. CSS file code: .both {clear:both;} .resourcetitle {font-size:2.25em; text-align:center; font-weight:bold;} .mainresources {width:80%; background-color:#f6f6f6; margin: 0 auto; } .mainresources2 {width:80%; background-color:#f6f6f6; margin: 0 auto;} .leftresource {float:left;} .rightresource {float:right;}
-
yes declare the var outside $(document).ready and that should do it: var myVar; $(document).ready(function(){ myVar = 1 echoMyVar(); }); function echoMyVar(){ alert(myVar); };
-
If it has an installation step, then put it on there. Depends on whether you're doing it username based or URL based license, so you can make a page on your host to receive a HTTP post with username/license code/url and check the data and HTTP post something back to the page where it came from. It's too dangerous to distribute a script with your database host/username/password on it.
-
this.each(function(i) { $($(this).attr('rel')).hide(); $(this).click(show); }); Above 4 lines in bold are hiding the images. I guess you could do a check to see if they're hidden already, if you're sure that's what's causing Opera to choke: this.each(function(i) { if( $($(this).attr('rel')).css("display") != "none"){ $($(this).attr('rel')).hide(); }; $(this).click(show); });
-
No problem, it's not so much about the attributes, i just chose "rel" because it validates and it wasn't being used by anything else I personally would use the "data" attribute but it won't validate but it will still work. Have a nice holiday too.
-
I think you forgot to include the image on the page (preferably wrapped with a div with a class AND and ID) and reference the ID of the <div> in the "rel" attribute of the link. (It's just like how you do it normally with Fancyzoom, but you need to put the ID of the div containing the large image in the "rel" attribute of the link instead of the "href") Try this: <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" lang="en"> <head> <script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.2.6/jquery.min.js"></script> <script type="text/javascript" src="js/fancyzoom2.js"></script> <script type="text/javascript" charset="utf-8"> $(document).ready(function() { $('div.photo a').fancyZoom({scaleImg: true, closeOnClick: true}); }); </script> <style type="text/css">.hideMe { display:none; }</style> </head> <body> <div class="photo"> <a href="../../images/family-photo.jpg" rel="#myPhotoDiv">TEST LINK</a> </div> <div class="hideMe" id="myPhotoDiv"> <img src="../../images/family-photo.jpg" alt="Family-Photo" /> </div> </body> </html>
-
Oh, and you need to hide the target divs (the ones with the large images) using CSS "display:none" so they don't show up when JS is off (fancyzoom.js does this automatically but the images show up when JS is off, which is not what we want)
-
Use this instead of the normal fancyzoom.js jQuery.fn.fancyZoom = function(options){ var options = options || {}; var directory = options && options.directory ? options.directory : 'images'; var zooming = false; if ($('#zoom').length == 0) { var ext = $.browser.msie ? 'gif' : 'png'; var html = '<div id="zoom" style="display:none;"> \ <table id="zoom_table" style="border-collapse:collapse; width:100%; height:100%;"> \ <tbody> \ <tr> \ <td class="tl" style="background:url(' + directory + '/tl.' + ext + ') 0 0 no-repeat; width:20px; height:20px; overflow:hidden;" /> \ <td class="tm" style="background:url(' + directory + '/tm.' + ext + ') 0 0 repeat-x; height:20px; overflow:hidden;" /> \ <td class="tr" style="background:url(' + directory + '/tr.' + ext + ') 100% 0 no-repeat; width:20px; height:20px; overflow:hidden;" /> \ </tr> \ <tr> \ <td class="ml" style="background:url(' + directory + '/ml.' + ext + ') 0 0 repeat-y; width:20px; overflow:hidden;" /> \ <td class="mm" style="background:#fff; vertical-align:top; padding:10px;"> \ <div id="zoom_content"> \ </div> \ </td> \ <td class="mr" style="background:url(' + directory + '/mr.' + ext + ') 100% 0 repeat-y; width:20px; overflow:hidden;" /> \ </tr> \ <tr> \ <td class="bl" style="background:url(' + directory + '/bl.' + ext + ') 0 100% no-repeat; width:20px; height:20px; overflow:hidden;" /> \ <td class="bm" style="background:url(' + directory + '/bm.' + ext + ') 0 100% repeat-x; height:20px; overflow:hidden;" /> \ <td class="br" style="background:url(' + directory + '/br.' + ext + ') 100% 100% no-repeat; width:20px; height:20px; overflow:hidden;" /> \ </tr> \ </tbody> \ </table> \ <a href="#" title="Close" id="zoom_close" style="position:absolute; top:0; left:0;"> \ <img src="' + directory + '/closebox.' + ext + '" alt="Close" style="border:none; margin:0; padding:0;" /> \ </a> \ </div>'; $('body').append(html); $('html').click(function(e){if($(e.target).parents('#zoom:visible').length == 0) hide();}); $(document).keyup(function(event){ if (event.keyCode == 27 && $('#zoom:visible').length > 0) hide(); }); $('#zoom_close').click(hide); } var zoom = $('#zoom'); var zoom_table = $('#zoom_table'); var zoom_close = $('#zoom_close'); var zoom_content = $('#zoom_content'); var middle_row = $('td.ml,td.mm,td.mr'); this.each(function(i) { $($(this).attr('rel')).hide(); $(this).click(show); }); return this; function show(e) { e.preventDefault(); if (zooming) return false; zooming = true; var content_div = $($(this).attr('rel')); var zoom_width = options.width; var zoom_height = options.height; var width = window.innerWidth || (window.document.documentElement.clientWidth || window.document.body.clientWidth); var height = window.innerHeight || (window.document.documentElement.clientHeight || window.document.body.clientHeight); var x = window.pageXOffset || (window.document.documentElement.scrollLeft || window.document.body.scrollLeft); var y = window.pageYOffset || (window.document.documentElement.scrollTop || window.document.body.scrollTop); var window_size = {'width':width, 'height':height, 'x':x, 'y':y} var width = (zoom_width || content_div.width()) + 60; var height = (zoom_height || content_div.height()) + 60; var d = window_size; // ensure that newTop is at least 0 so it doesn't hide close button var newTop = Math.max((d.height/2) - (height/2) + y, 0); var newLeft = (d.width/2) - (width/2); var curTop = e.pageY; var curLeft = e.pageX; zoom_close.attr('curTop', curTop); zoom_close.attr('curLeft', curLeft); zoom_close.attr('scaleImg', options.scaleImg ? 'true' : 'false'); $('#zoom').hide().css({ position : 'absolute', top : curTop + 'px', left : curLeft + 'px', width : '1px', height : '1px' }); fixBackgroundsForIE(); zoom_close.hide(); if (options.closeOnClick) { $('#zoom').click(hide); } if (options.scaleImg) { zoom_content.html(content_div.html()); $('#zoom_content img').css('width', '100%'); } else { zoom_content.html(''); } $('#zoom').animate({ top : newTop + 'px', left : newLeft + 'px', opacity : "show", width : width, height : height }, 500, null, function() { if (options.scaleImg != true) { zoom_content.html(content_div.html()); } unfixBackgroundsForIE(); zoom_close.show(); zooming = false; }) return false; } function hide() { if (zooming) return false; zooming = true; $('#zoom').unbind('click'); fixBackgroundsForIE(); if (zoom_close.attr('scaleImg') != 'true') { zoom_content.html(''); } zoom_close.hide(); $('#zoom').animate({ top : zoom_close.attr('curTop') + 'px', left : zoom_close.attr('curLeft') + 'px', opacity : "hide", width : '1px', height : '1px' }, 500, null, function() { if (zoom_close.attr('scaleImg') == 'true') { zoom_content.html(''); } unfixBackgroundsForIE(); zooming = false; }); return false; } function switchBackgroundImagesTo(to) { $('#zoom_table td').each(function(i) { var bg = $(this).css('background-image').replace(/\.(png|gif|none)\"\)$/, '.' + to + '")'); $(this).css('background-image', bg); }); var close_img = zoom_close.children('img'); var new_img = close_img.attr('src').replace(/\.(png|gif|none)$/, '.' + to); close_img.attr('src', new_img); } function fixBackgroundsForIE() { if ($.browser.msie && parseFloat($.browser.version) >= 7) { switchBackgroundImagesTo('gif'); } } function unfixBackgroundsForIE() { if ($.browser.msie && $.browser.version >= 7) { switchBackgroundImagesTo('png'); } } } I changed it so it works with the "rel" attribute instead of the "href" Just drop the target ID (example: #github) inside the "rel" attribute instead of the "href" and drop the actual URL to the image inside the href attribute so it links straight to the image when JS is off. I haven't tested this but it should work. If it doesn't then can u post any Javascript error you get (preferably from Firebug instead of IE Javascript errors lol)
-
http://fancybox.net/ I tried this one without Javascript, and it just shows the thumbnails, when you click on them they just link to the image itself. It's quite fast too, but the thing with this one is from time to time, when you click on the thumbnail, it displays backlinks to the author's website, you need to buy a license to disable that feature I think.
-
Have you tried using another variable? like $q or $j or $v or something like that
-
Isn't it just the scrollbar? Also, Firebug shows what the browser has interpreted, so Firefox etc auto-close unclosed tags, but in View Source it will show the original code.
-
Think it automatically skips the animation, and puts the element into the state it's supposed to be in, instead of cancelling the animation halfway. But yea, what Ben said is pretty much the case for animation queue. It's quite standard these days, as it's not very practical for the menu to be animating itself even after the mouse has stopped moving.
-
http://www.w3schools.com Good place to start.
-
Your script runs fine to me. But it's running only after all images have loaded. Did you see your "Loading" bar? window.addEventListener?window.addEventListener("load",so_init,false):window.attachEvent("onload",so_init); This line makes your fade thing run when the page has "loaded". So that means it waits for all the images and all other stuff to be fully loaded until it runs.
-
I'm not familiar with the Killersites shopping cart system, but looks like the questions are more about Paypal, so I'll have a go: Question 1: Yes it's quite possible. You just need to add "sandbox" to the address on your IPN handler page. The IPN handler page first receives a POST data from paypal, then it should send the data back to paypal to verify, you just need to change the address it sends the verification to: https://www.sandbox.paypal.com/cgi-bin/webscr or something like that. Question 2: I'm not really up to date with the evolving API of paypal, but from what I can remember, you can pass few different variables to paypal. There's one called "custom": <input type="hidden" name="custom" value="<!--Your Member ID Value Here-->" /> And from what I remember from a recent paypal site I made, this "custom" variable comes back from paypal to your website via IPN and also PDT. There's another way to add custom variables to paypal, and those variables called on_0 os_0, on_1 os_1, on_2 os_2 ... "on" stands for "option name" and os stands for "option selection" or something. Basically you must put the variable name in "on" and the value in "os" (So they must be in pairs basically): <input type="hidden" name="on_0" value="member_id" /> <input type="hidden" name="os_0" value="000123456" /> These might not be 100% accurate, and might be outdated so you should check the Paypal PDF's just to make sure
-
SEO is a very broad field. There's so many things involved, and they all work together to contribute to your search engine ranking. Things like (in no particular order): - Good quality Inbound links, from high ranked websites (a link from BBC.co.uk will greatly boost your rankings) - Non-duplicated Title tags for your pages, and also non-duplicate "description" meta tag - Content-relevant "Description" meta tags - Having content at the top of the HTML structure - Having only one (and at least one) "h1" tag on the page, and use h2, h3 for other headings (as many as u want on a page). - Having Semantic HTML (<h1>About Us</h1> is semantic, instead of <span class="heading1">About Us</span> which is not very semantic) - Speeding up your page loading times (This is a very broad field too...) - Submitting and confirming your site on "Google Webmaster Tools" and also submitting your XML sitemap there so Googlebot knows which pages you have on your website. (Unless you haven't already done so) - Submitting your site to other search engines (Unless you haven't already done so) - Most important: Having unique content, and updating your site regularly with new unique content, and don't forget to update your sitemap on Google Webmaster Tools after you create new content. ... and much more. Don't mean to overwhelm you though, it's just the way it is So start small, and work your way up, and don't take shortcuts, learn things properly from the beginning and you will set a good foundation for yourself to work on. Good luck.
-
You can try "Lazy Loading" technique. There's few jQuery plugins out there, but I'm not 100% sure that it will work well with Lightbox/Hidden images. Or if worse comes to worst, you can just "Pre-load" the images in the slideshows Then when people click on the images, the images inside the lightbox will come up instantly.
-
Yea I do it this way all the time. Important thing to remember though is to: Disallow: /folderName/ in robots.txt which should sit in the root folder of your website.
-
A more elegant approach is to use defaultValue property. That way, if you change the value text, you don't need to go and update the onclick event script as well... <form method="get" class="searchform" action="http://www.website.com/" > <input type="text" value="Search this website" name="s" class="s" onfocus="if (this.value == this.defaultValue) {this.value = '';}" onblur="if (this.value == '') {this.value = this.defaultValue;}" /> <input type="submit" class="searchsubmit" value="Search" /> </form>
-
Just think if ID's as things that can overrule Class css rules. It's especially useful when you have a CMS system, and you don't have much control over the Classes and ID's that the CMS generates, however you have total control over the CSS document. And CMS systems tend to use same classes for many different things. To really understand the usefulness of ID's and Classes working together, you need to read up a bit on "CSS Specificity" I think, because that's where the real usefulness of ID's comes shining through.
-
No not really. CSS rule specified by an ID selector will overrule a CSS rule specified by a Class selector. <div id="box1" class="square"></div> #box1 { width:100px; } .square { width:50000px; } Above div will have a width of 100px; You mean must all have different name? ID's and classes both serve different purposes. If you wish to style a group of things similarly, then you use Classes, if you want to style an individual item out of a group of similarly styled items, then you put an ID on it and set its CSS rule. ID's are even more useful when using Javascript/jQuery
-
Yeah, basically you don't need ID's on the <li> or the <a> inside them. May be a good idea to put a same "class" on the <a> so you can exclusively select those anchors. There's lots of ways to approach this though, there's no single correct way. Say you give those <a>'s a class="trigger". <ul> <li><a href="#birthday" class="trigger">Birthdays</a></li> ..... ..... </ul> <div id="div_container"> <div id="birthday"> ..... </div> <div id="...."> ..... </div> ..... </div> jQuery(function($){ var $trigs = $("a.trigger"); var $cont = $("#div_container"); var $all = $("div", $cont); // Selects all descendant <div> of $cont $trigs.click(function(e){ // Cancel default link action so the // browser won't jump to top e.preventDefault(); // Get the ID of the target div which is // in the "href" attribute of the clicked anchor var $target = $(this).attr("href"); // fadeOut all the divs first $all.fadeOut(250); // fadeIn the target $( $target ).fadeIn(250); }); });
-
Normal Javascript shouldn't create that message. Without looking at the page I can't tell you much about this. If you have an online draft page then post a link here. Can also have a look in terms of performance issues etc you mentioned on the original post.