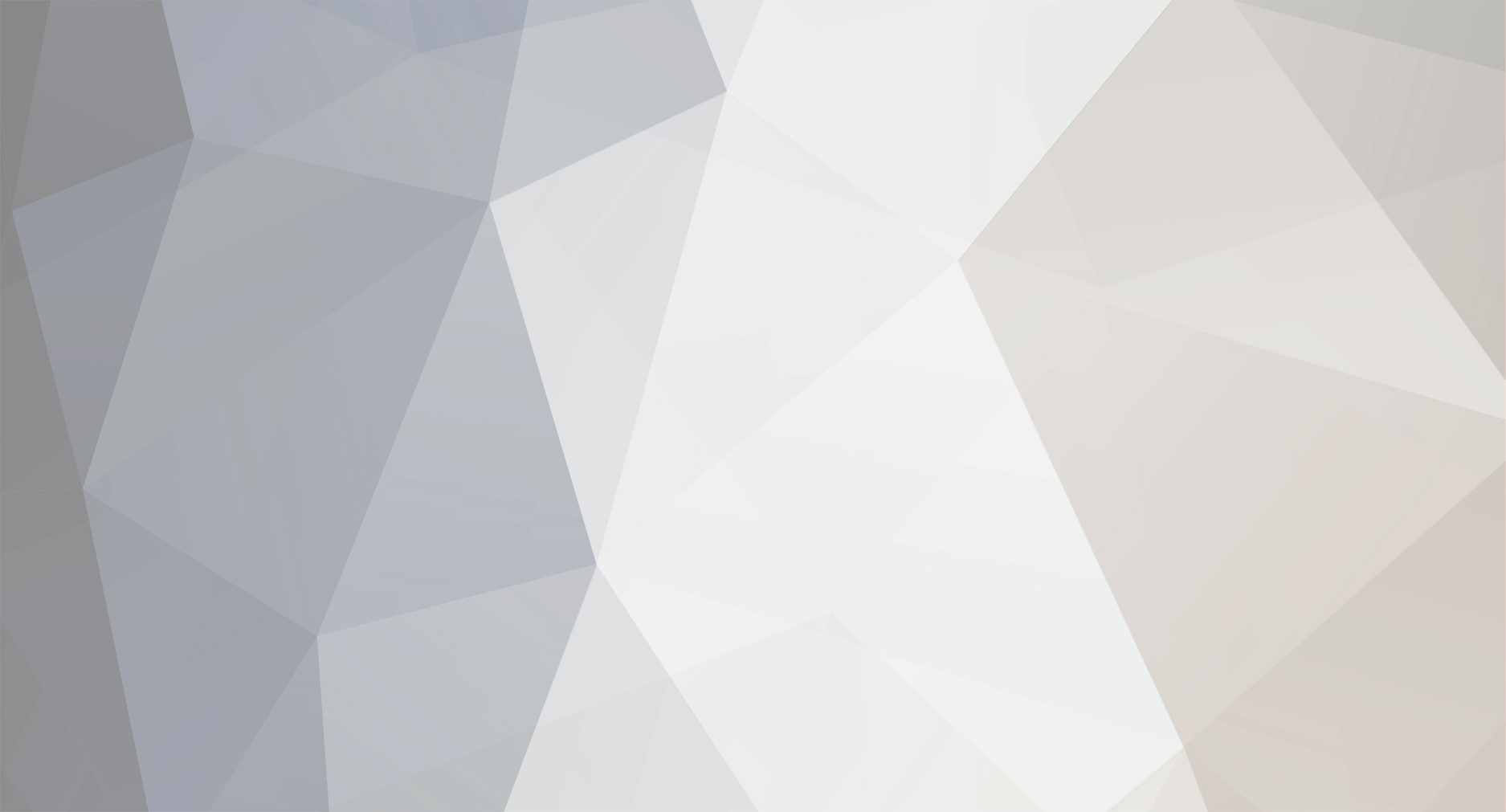
gregan
Member-
Posts
8 -
Joined
-
Last visited
gregan's Achievements
Newbie (1/14)
0
Reputation
-
Sorry about the thread kind of evolving as I continue to develop off of the tutorial of the OOP Login. I was successful in the process of setting the sessions for a id, username, and level. I figured I would post the code for it that I have below. While working on this, I realized that it may be most efficient to create class variables(var $id, var $username, var $email, & var $userlevel, etc...) in my User class (m_user.php) and set them whenever a user is logged in or out. So that I can easily access the currently logged in User's information while in the User class with $this-> rather then accessing the SESSION everytime I need the id or say... LastName of the currently logged in user. Just putting my thoughts out there as I work on expanding this CMS a bit to improve on managing the users & userlevels, typing it out helps me think about it. edit: as well as possibly being an easier way to track active users & active guests. function validateLogin($user, $pass) { global $Database; if($stmt = $Database->prepare("SELECT id, username, userlevel, email FROM ". TBL_USERS ." WHERE username = ? AND password = ?")) { $stmt->bind_param("ss", $user, md5($pass . $this->salt)); $stmt->execute(); $stmt->store_result(); $stmt->bind_result($id, $username, $userlevel, $email); //Logged in, or Not Logged In if($stmt->num_rows > 0) { while($stmt->fetch()) { $_SESSION['loggedin'] = TRUE; $_SESSION['id'] = $id; $_SESSION['username'] = $username; $_SESSION['userlevel'] = $userlevel; $_SESSION['email'] = $email; } $stmt->close(); return TRUE; } else { $stmt->close(); $_SESSION['username'] = GUEST_NAME; $_SESSION['userlevel'] = GUEST_LEVEL; return FALSE; } } else { die("ERROR: Could not prepare MySQLi statement."); } }
-
Ben, I hope to purchase this in the near future, looks like it could help me a lot... Thanks I was successful in getting the getUserInfo($id) class to work successfully, I have now run into another challenge while creating a page for a unique id. I am trying to figure out how I can figure out a way to know if the $id being viewed is the $id of the user currently logged in. $Template->setData('loggedin', $Auth->checkLoginStatus(), false); I am able to get the login status, although not the logged in id. I am thinking the best way to perform this would be to set a $_SESSION['id'], in the validateLogin function? And then check if $_GET['id'] == $_SESSION['id']? Also most likely would be useful to store username and userlevel into a $_SESSION? Thanks Greg
-
Ughhhh, stupid mistake by me on the is_numeric. Everything works correctly after I fixed that. Thanks you really assisted me a lot in this process. Is your "build a CMS" MVC OOP? I am interested and knowing a bit more about this video course. http://www.killervideostore.com/video-courses/build-a-cms.php
-
Hey, I attempted your suggestion and am not getting any success. I am not even sure if I am calling my array correctly in the 3rd code box, but it does not seem to be storing anything at all in $rows. Any advice would be appreciated. Greg function getUserInfo($id) { global $Database; $stmt = $Database->prepare("SELECT username, userlevel, email FROM ". TBL_USERS ." WHERE id = ?"); $stmt->bind_param('i', is_numeric($id)); $results = $stmt->execute(); $stmt->store_result(); $stmt->bind_result($username, $userlevel, $email); $rows = array(); while ($stmt->fetch()) { $newrow = array(); $newrow['id'] = $id; $newrow['username'] = $username; $newrow['userlevel'] = $userlevel; $newrow['email'] = $email; $rows[] = $newrow; } if (count($rows) == 0) { return false; } else { return $rows; } } $Template->setData('query', $User->getUserInfo(2), false); if ($result = $this->getData('query')) { echo $result[0]['username']; }
-
One more question... If I want to bind a value first before returning the query... How do I successfully return this? Thanks function getUserInfo($id) { global $Database; $stmt = $Database->query("SELECT * FROM ". TBL_USERS ." WHERE id = ?"); $stmt->bind_param("i", $id); $stmt->execute(); return $stmt; }
-
Hey thanks so much for helping me understand the process. I have made the changes and have it successfully working. I have personally decided to keep the loop in the view for now just for personal preference. I understand why it may be better to have in the controller, I just feel that some pages may become to complex to continue to do this. For example, a homepage that may have to show recent posts, recent members, recent photos, etc... I think it may get a bit too complex in the controller and feel the loop would be better in the view. Here is my final code for reference for my files. <?php include("includes/database.php"); include("includes/init.php"); $Template->setData('query', $Users->getAllUsers(), false); $Template->load("views/v_members.php"); <div class="row"> <?php if ($result = $this->getData('query')) { if ($result->num_rows > 0) { echo "<table border='1' cellpadding='10'>"; echo "<tr><th>ID</th><th>Username</th><th>Email</th><th>User Level</th><th></th><th></th></tr>"; while ($row = $result->fetch_object()) { echo "<tr>"; echo "<td>" . $row->id . "</td>"; echo "<td>" . $row->username . "</td>"; echo "<td>" . $row->email . "</td>"; echo "<td>" . $row->userlevel . "</td>"; echo "<td><a href='records.php?id=" . $row->id . "'>Edit</a></td>"; echo "<td><a href='delete.php?id=" . $row->id . "'>Delete</a></td>"; echo "</tr>"; } echo "</table>"; } else { echo "No results to display!"; } } ?> </div> function setData($name, $value, $clean = TRUE) { if($clean) { $this->data[$name] = htmlentities($value); } else { $this->data[$name] = $value; } } class Users { /* Constructor */ function __construct() { } /* Functions */ function getAllUsers() { global $Database; $results = $Database->query("SELECT id, username, userlevel, email FROM ". TBL_USERS .""); return $results; } }
-
Hey thanks for the great response... Below is my attempt at following your suggestion. Although I have run into error while running the setData on the query(), which is because it's looking for a string. edit: Also, is it correct having the loop occur in the view? I just began thinking about having the loop in the controller, then each time through set somehow set a two d array or use the setData in a loop? $data->setData['username'] = $row->username; I am not sure how to do get them in the view though, inside another loop? members.php <?php include("includes/database.php"); include("includes/init.php"); $Template->setData('query', $Users->getAllUsers()); $Template->load("views/v_members.php"); v_members.php <?php if ($result = $this->getData['query']) { // display records if there are records to display if ($result->num_rows > 0) { // display records in a table echo "<table border='1' cellpadding='10'>"; // set table headers echo "<tr><th>ID</th><th>Username</th><th>Email</th><th>User Level</th><th></th><th></th></tr>"; while ($row = $result->fetch_object()) { // set up a row for each record echo "<tr>"; echo "<td>" . $row->id . "</td>"; echo "<td>" . $row->username . "</td>"; echo "<td>" . $row->email . "</td>"; echo "<td>" . $row->userlevel . "</td>"; echo "<td><a href='records.php?id=" . $row->id . "'>Edit</a></td>"; echo "<td><a href='delete.php?id=" . $row->id . "'>Delete</a></td>"; echo "</tr>"; } echo "</table>"; } // if there are no records in the database, display an alert message else { echo "No results to display!"; } } ?> m_users.php <?php /* Users Class */ class Users { /* Constructor */ function __construct() { } /* Functions */ function getAllUsers() { global $Database; $results = $Database->query("SELECT id, username, userlevel, email FROM ". TBL_USERS .""); return $results; } }
-
Hey guys, New to the forums. Came for some help and a better understanding! So I have completed and watched the entire OOP Login tutorial and I have began trying to implement my own features. To avoid confusion - I have renamed the tutorial's members/v_members to main/v_main.. And am using the members/v_members for this users page. I am working on creating a members page where you can see all of the registered users. My issue is with accessing the database and how I should be doing this. a) How can I successfully complete this task and cleanup my code? $stmt = "SELECT id, username, email, userlevel FROM users"; global $Database; Should this line be in v_members or members? c) I think my issue is with my foreach loop, but I am not sure how to correct this. members.php <?php include("includes/database.php"); include("includes/init.php"); $Template->load("views/v_members.php"); v_members.php <div> <?php $alerts = $this->getAlerts(); if ($alerts != '') { echo '<ul class="alerts">' . $alerts . '</ul>'; } ?> <?php //Get users $stmt = "SELECT id, username, email, userlevel FROM users"; global $Database; ?> <div class="row"> <?php foreach ($Database->query($stmt) as $row) { ?> ID: <?php echo $row['id']; ?> <br /> User: <?php echo $row['username']; ?> <br /> Email: <?php echo $row['email']; ?> <br /> <?php } ?> </div> My page results are as follows. I only have 1 entry in the database. ID: User: Email: ID: User: Email: ID: User: Email: ID: User: Email: ID: User: Email: