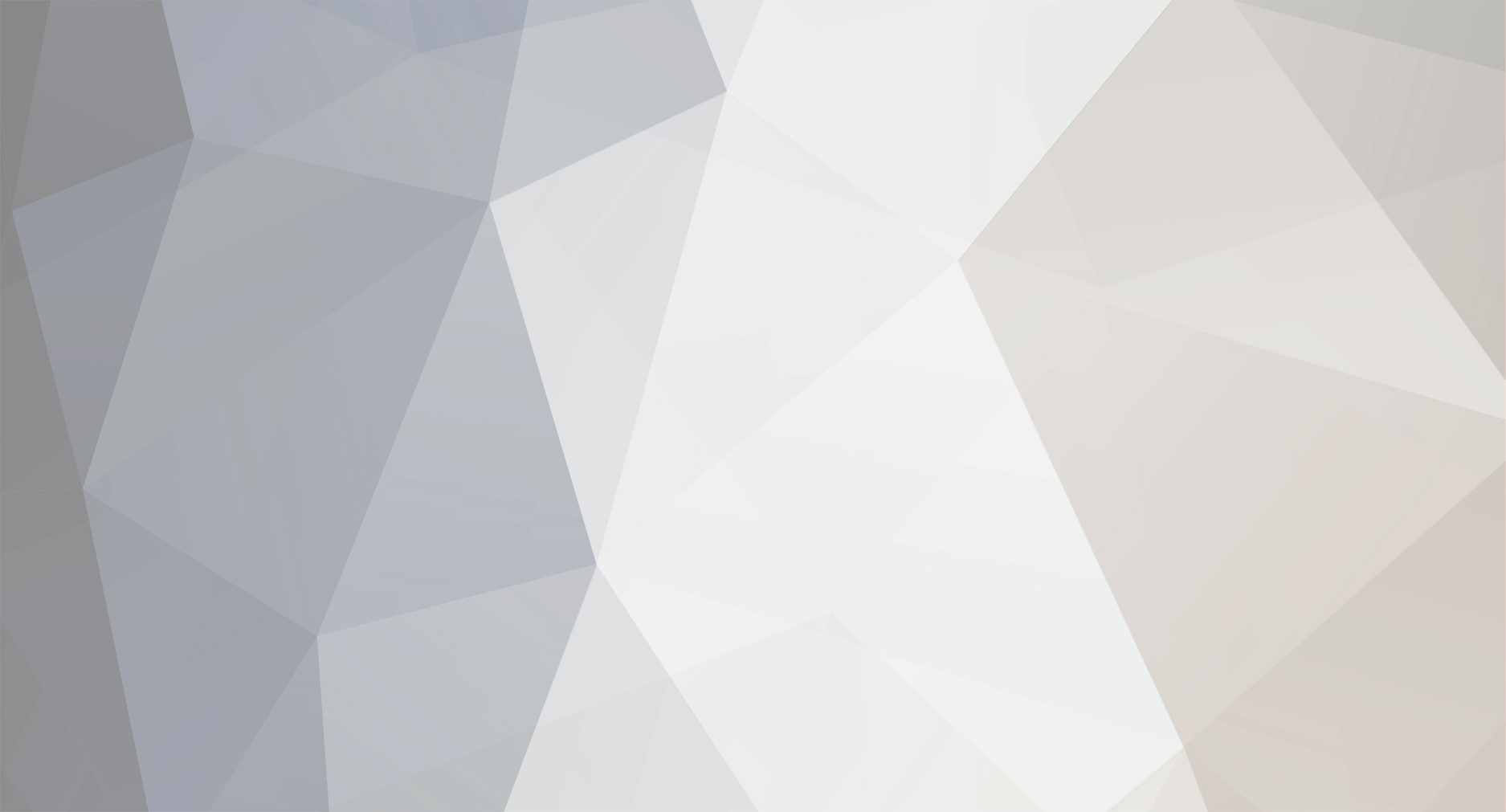
is_numeric
-
Posts
21 -
Joined
-
Last visited
Content Type
Profiles
Forums
Events
Downloads
Gallery
Store
Posts posted by is_numeric
-
-
If you have access to the mail boxes then you could forward mail from these to your primary email address or the companies could add you as CC or BCC.
-
I thought it was bad practice to globalise objects?
Cant you just pass in the object to the class as an argument or instantiate the object from within the other class?
-
I have been using notepad++ for a few months now and its brilliant. Very light weight and fast. It has its own FTP client and file explorer. Syntax colouring and loads more plugins. Its ideal for making quick changes as well. Also try the Zen Coding plugin. This provides an HTML template code for quick HTML implimentation.
For example...
html>(head>title)>body>div#wrapper>div#header+div#left+div#content+div#footer
produces...
<html> <head> <title></title> </head> <body> <div id="wrapper"> <div id="header"></div> <div id="left"></div> <div id="content"></div> <div id="footer"></div> </div> </body> </html>
And
ul>li#list$*5
produces...
<ul> <li id="list1"></li> <li id="list2"></li> <li id="list3"></li> <li id="list4"></li> <li id="list5"></li> </ul>
Mint!
-
This is Dreamweaver produced code. If we hack it then the risk is you wont be able to edit it in the DW panels and therefore put you in a worse position than you are in now
-
PHP has a PDF library built in
http://php.net/manual/en/book.pdf.php
although word on the street say this is a better option
-
Note the way I have written the array and then looped through it performing the change
<?php $array = array( "category1\ncategory2\ncategory3\ncategory4", "category1\ncategory2\ncategory3\ncategory4\ncategory5" ); foreach($array as $values){ $newArray[] = preg_replace("(\n)",",",$values); } echo "<pre>"; print_r($newArray); echo "</pre>"; ?>
-
Hi all
I provide a web development training service for noobs
My stats are telling me I get loads of visits but I am only converting a handful of sales a month
Any tips around converting leads would be great
The site is here
-
You can do this a number of ways using PHP...
Look at fwrite
[example]...
$f = fopen('/tmp/data.csv', 'a'); //<< in 'a' append mode fwrite($f, 'line of data 1'); //<< you will have to loop through this line fclose($f); // close handle
Or using a MYSQL query...
[example]...
SELECT field1,field2,field3 FROM table INTO OUTFILE '/tmp/data.csv' FIELDS TERMINATED BY ',' ENCLOSED BY '"' LINES TERMINATED BY '\n'
-
I would ditch the mysqli method in favour of PDO.
Its the way forward
-
I suggest using Drupal CMS for your project
you can use the Ubercart module and build a nice ecomm site which will handle memberships and payments. Ubercart has payment gateway interfaces so is quite nice.
It will be a steep learning curve though.
I am available for paid consultancy if you need a hand
-
Gotcha
Thanks again
-
Thanks Krillz
I didnt think you needed a destructor since PHP 5 as the objects are disposed of automatically
-
In the library example where do you instantiate the object?
-
ok
say I had the following classes
QueryClass //<< Runs SQL and query databases LoginClass //<< Does what it says FormProcessClass //<< cleans form data DataFormatterClass //<< Takes query output and creates a Pagination table
If I want to access a method from one class to another and possibly another what's the preferred way
Example (This is a made up example)
I instantiate my FormProcessClass and pass in the $_POST'ed data from a form for cleaning and then return the output.
I then need to pass this into my QueryClass which does a db SELECT maybe
If the QueryClass returns a result from the SELECT statement then use this output in in my DataFormatterClass and return a nice table of data
Do I pass in the objects like this making them available to all other methods
$class1 = new FormProcessClass($_POST); $class2 = new QueryClass($class1); $class3 = new DataFormatterClass($class1,$class2);
-
Bought it... Well work did
Really cool
-
contact me regarding your problem here
I need to understand it better
-
cant you just cast the type to what you want
$newVar = (int)$var;
-
If you understand OO PHP then you will know not to code anything which will render your efforts bespoke
getters and setters and an interface will be your best way here.
-
I agree with many points here and a lot of programmers code in OO style and forget why they do it. As its been said abstraction and modular development with re-usability the driving factor but these things get lost in an undisciplined environment. How many apps have we written where we have recoded our connection methods or query methods time and time again. I too fall into this trap way too often. If we were to plan an application properly we could start creating a suite of classes which theoretically could live above all our project directories and each project share each class as and when needed.
Pulling one web site into another
in PHP
Posted
The best way would be to develop a Web Service which you would send the content of the catalogue back as XML. This would allow the developer at the other end to theme and style the content accordingly and provide more flexibility.