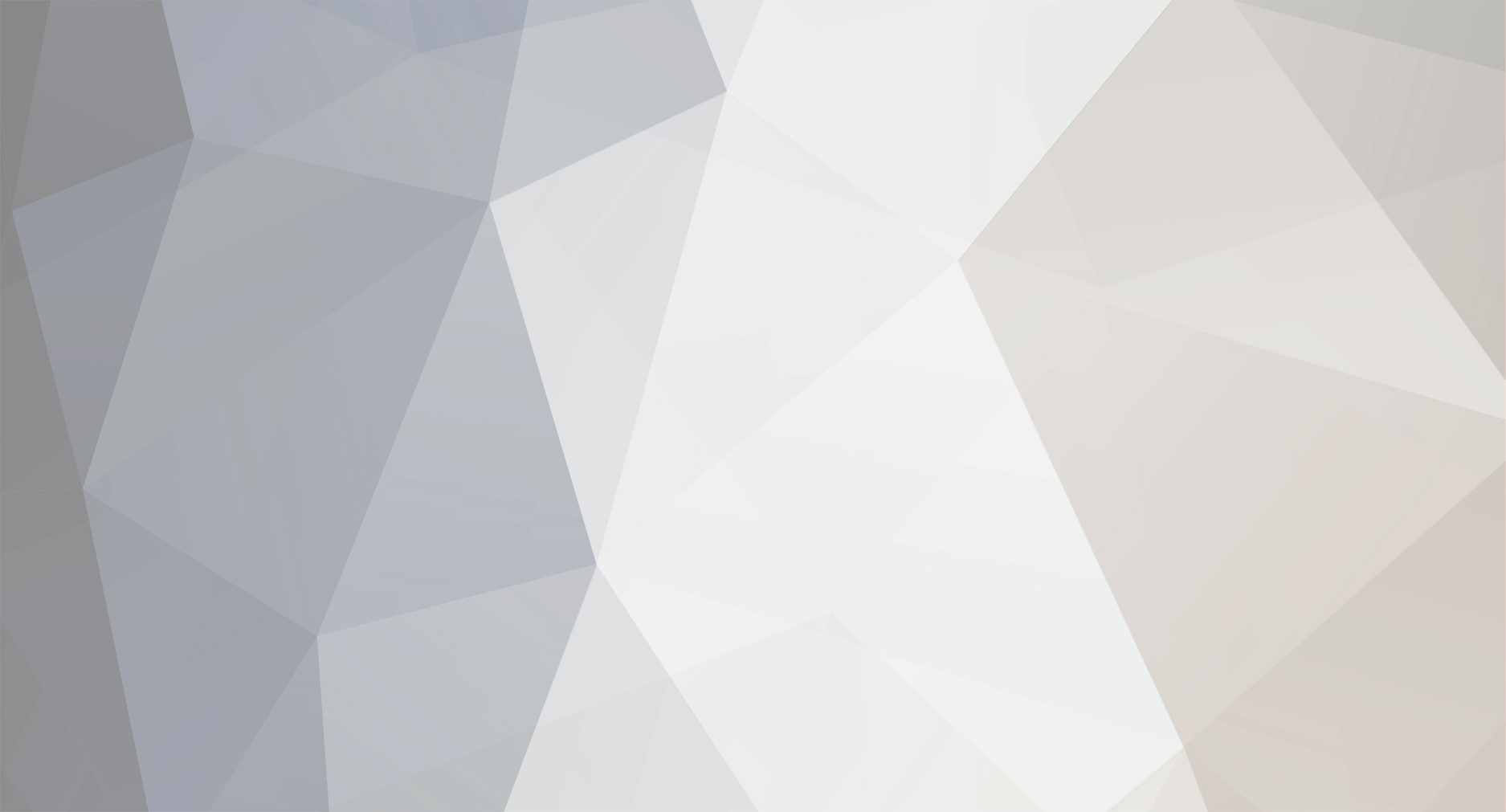
Johnny2
-
Posts
52 -
Joined
-
Last visited
Content Type
Profiles
Forums
Events
Downloads
Gallery
Store
Posts posted by Johnny2
-
-
Are you doing this with AJAX or something? Why the long load time? If you're using header() to redirect, that redirect should be instantaneous (thought the page the user gets redirected to may show a blank white page as it loads).
...I think the bigger question here is why your page is taking 20+ seconds to load though. Loading page or not, you actually expect people to wait around for that? I wouldn't.
It's not always 21 seconds. It can be as low as 2 seconds if a bunch of info doesn't have to be gotten from databases for the particular user. But for the users that need more information gathered, I wanted a loading message to display.
-
Right when my user logs in, the login.php file sends them to another file (we'll call it File_2) which takes about 21 seconds to load... so the user is staring at the filled-in login form for 21 seconds before they see anything else happen.
After the user clicks the login button, I'd like to display something to the user which indicates the page is loading.
At the end of "File_2", after it is all finished gathering data, it displays the html.
I'm having difficulty figuring out how to display a "loading" message while the user waits. Any help would be appreciated.
I've tried having the user sent from the login.php file to "File_1" THEN to "File_2", but this didn't work. It had the same effect as if the user was sent from login.php directly to "File_2".
Code I've used:
In login.php file:
// After the user is granted permission to login: header("Location: File_1.php"); exit;
File_1 (where I was trying to show the user "Page is Loading", while they waited:
<?php session_start(); ?> <!doctype html> <html> <head> <meta charset="utf-8"> <title>Loading</title> </head> <body> <h1>The Page is Loading. This may take a few moments.</h1> </body> </html> <?php header("Location: File_2.php"); exit; ?>
Then File_2 is just the page that loads, but the user doesn't see anything happen for 21 seconds.
-
I'm using the following code to save a file from a form:
<?php $item_ID=53; // ADDS IMAGES TO IMAGES FOLDER $image_name_1 = "$item_ID"."_img1".".jpg"; //Saves newly-named image file move_uploaded_file($_FILES['img1']['tmp_name'],"../images/$image_name_1"); ?>
The code works great.
However; some files become turned on their side when viewed on the website.
I'm taking a shot in the dark and thinking it has something to do with the way Windows 8 saves the file. Because if I take the same file and re-save it in photoshop, then upload that file, it is not turned on it's side.
Anyway, I cannot alter the code of windows, so is there some php code that I can use to make sure the image isn't turned on it's side when uploaded via my script?
Thanks.
-
-
Thank you for your help!
-
Is there a quick and easy way to get a webpage's banner image to load BEFORE the webpage displays itself to the browser?
I have a page that displays, then a quarter of a second later the main image displays itself. This causes things to jump around and makes it seem a little glitchy.
Thanks.
-
Hello,
I can't figure out a few lines of cURL code.
I have 3 files involved...
File #1 will have a form that submits the form data (via POST) to FILE #2.
File #2 receives the POST, automatically re-posts the exact same data to File #3, then continues to process some of the info the POST contained.
File #3 receives the POST from File #2, and processes it normally (and this is where the USER should end up).
What I can't figure out is the part (in file #2) that receives the POST then AUTOMATICALLY REPOSTS it. The cURL code that I have to work with doesn't seem to work so far. Here's what I have to work with:
$url='File_number_3'; //open connection $ch = curl_init(); //set the url, number of POST vars, POST data curl_setopt($ch,CURLOPT_URL,$url); curl_setopt($ch,CURLOPT_POST,count($_POST)); curl_setopt($ch,CURLOPT_POSTFIELDS,$_POST); //execute post $result = curl_exec($ch); //close connection curl_close($ch);
Anyone know what I need to do with this code to simply get it to take the incoming POST, then re-submit it to File_number_3 ?
Thanks
-
I have this multidimensional array that I'll call "$Items_Array":
array (size=3) 0 => array (size=3) 'id' => string '1' (length=1) 'quantity' => int 1 'name' => string 'Book' (length=1) 1 => array (size=3) 'id' => string '2' (length=1) 'quantity' => int 5 'name' => string 'Shirt' (length=29) 2 => array (size=3) 'id' => string '3' (length=1) 'quantity' => int 2 'name' => string 'Ice-cream' (length=22)
I want to re-arrange the order of elements, according to "quantity", so that the one with the largest quantity comes first. The desired result would look like this:
array (size=3) 0 => array (size=3) 'id' => string '2' (length=1) 'quantity' => int 5 'name' => string 'Shirt' (length=29) 1 => array (size=3) 'id' => string '3' (length=1) 'quantity' => int 2 'name' => string 'Ice-cream' (length=22) 2 => array (size=3) 'id' => string '1' (length=1) 'quantity' => int 1 'name' => string 'Book' (length=1)
Can someone show me how to do this? Is it just an easy PHP command?
-
I have 2 questions about the basic understanding of website security.
#1: Once I have my website built, get it hosted, and I'm ready to go live, how do I go about making sure my website Security is up to par? Are there website security companies that try to hack into your site and find security holes, then report to you what you need to fix? At that point, how do I get the repairs done if I'm a non-expert?
#2: How do I become PCI Compliant (so I can take credit cards and whatnot)? Do I just call up some PCI Compliance company and have them analyze my website, then they tell me what to fix?
At that point, how do I get the repairs done if I'm a non-expert?
Thanks
-
Thanks for your input Eddie.
Let's say your website files & folders are supposed to go inside this made-up directory/folder:
123.456.78.90/public_html (this is kind of what the directory looks like when I'm in my c-panel anyways)
Are you saying that I should put most of my website files in this public_html folder, but put data I want to protect into a different folder which is on the same level as the public_html folder? Like here:
123.456.78.90/_data
If I'm correct, would you put files such as config files here (which may contain password info)?
Question #2:
How does this relate to session info (like session variables that I don't want hackers to get their hands on)?
-
Hi All,
I've come across some PHP website-building literature that stated:
"For sensitive data being stored, but not stored in a database, change your sessions directory, and use the Web root directory's parent folder"
I understand that no one here is the author, but could someone take a guess at and explain what this means? I'm not sure what a sessions directory is.
When it refers to "sessions", is it talking about session variables that we can create?... like if I wanted to store the logged-in user's first name in $_SESSION['userFirstName']?
Is temporarily storing potentially sensitive data in session variables not secure?
I'm pretty new at this, so please use plenty of laymen terms
Thank you so much!
-
Thanks Ben. Do you know of any tutorial videos on parsing XML or API's?
-
I was just wondering if I could get a short & sweet explanation for how to exchange data with other websites.
A common example would be requesting the shipping-cost for a package from UPS.
I'm pretty fuzzy with how one "catches" the data replied to you (from UPS), and how you would get that data put into a PHP variable. Once this "answer" to my request is in a PHP variable, I would be able to figure it out from there.
Their example code for a request:
<?xml version="1.0"?> <AccessRequest xml:lang="en-US"> <AccessLicenseNumber>Your_License</AccessLicenseNumber> <UserId>Your_ID</UserId> <Password>Your_Password</Password> </AccessRequest> <?xml version="1.0"?> <RatingServiceSelectionRequest xml:lang="en-US"> <Request> <TransactionReference> <CustomerContext>Rating and Service</CustomerContext> <XpciVersion>1.0</XpciVersion> </TransactionReference> <RequestAction>Rate</RequestAction> <RequestOption>Rate</RequestOption> </Request> <PickupType> <code>07</code> <Description>Rate</Description> </PickupType> <Shipment> <Description>Rate Description</Description> <Shipper> <Name>Name</Name> <PhoneNumber>1234567890</PhoneNumber> <ShipperNumber>Ship Number</ShipperNumber> <address> <AddressLine1>Address Line</AddressLine1> <City>City</City> <StateProvinceCode>NJ</StateProvinceCode> <PostalCode>07430</PostalCode> <CountryCode>US</CountryCode> </address> </Shipper> <ShipTo> <CompanyName>Company Name</CompanyName> <PhoneNumber>1234567890</PhoneNumber> <address> <AddressLine1>Address Line</AddressLine1> <City>Corado</City> <PostalCode>00646</PostalCode> <CountryCode>PR</CountryCode> </address> </ShipTo> <ShipFrom> <CompanyName>Company Name</CompanyName> <AttentionName>Attention Name</AttentionName> <PhoneNumber>1234567890</PhoneNumber> <FaxNumber>1234567890</FaxNumber> <address> <AddressLine1>Address Line</AddressLine1> <City>Boca Raton</City> <StateProvinceCode>FL</StateProvinceCode> <PostalCode>33434</PostalCode> <CountryCode>US</CountryCode> </address> </ShipFrom> <Service> <code>03</code> </Service> <PaymentInformation> <Prepaid> <BillShipper> <AccountNumber>Ship Number</AccountNumber> </BillShipper> </Prepaid> </PaymentInformation> <Package> <PackagingType> <code>02</code> <Description>Customer Supplied</Description> </PackagingType> <Description>Rate</Description> <PackageWeight> <UnitOfMeasurement> <code>LBS</code> </UnitOfMeasurement> <Weight>10</Weight> </PackageWeight> </Package> <ShipmentServiceOptions> <OnCallAir> <Schedule> <PickupDay>02</PickupDay> <Method>02</Method> </Schedule> </OnCallAir> </ShipmentServiceOptions> </Shipment> </RatingServiceSelectionRequest>
Their example of a Response (I have no idea how I would even get or work with this)
<?xml version="1.0" encoding="ISO-8859-1"?> <RatingServiceSelectionResponse> <Response> <TransactionReference> <CustomerContext>Rating and Service</CustomerContext> <XpciVersion>1.0</XpciVersion> </TransactionReference> <ResponseStatusCode>1</ResponseStatusCode> <ResponseStatusDescription>Success</ResponseStatusDescription> </Response> <RatedShipment> <Service> <Code>03</Code> </Service> <RatedShipmentWarning>Your invoice may vary from the displayed reference rates</RatedShipmentWarning> <BillingWeight> <UnitOfMeasurement> <Code>LBS</Code> </UnitOfMeasurement> <Weight>10.0</Weight> </BillingWeight> <TransportationCharges> <CurrencyCode>USD</CurrencyCode> <MonetaryValue>52.16</MonetaryValue> </TransportationCharges> <ServiceOptionsCharges> <CurrencyCode>USD</CurrencyCode> <MonetaryValue>0.00</MonetaryValue> </ServiceOptionsCharges> <TotalCharges> <CurrencyCode>USD</CurrencyCode> <MonetaryValue>52.16</MonetaryValue> </TotalCharges> <GuaranteedDaysToDelivery/> <ScheduledDeliveryTime/> <RatedPackage> <TransportationCharges> <CurrencyCode>USD</CurrencyCode> <MonetaryValue>52.16</MonetaryValue> </TransportationCharges> <ServiceOptionsCharges> <CurrencyCode>USD</CurrencyCode> <MonetaryValue>0.00</MonetaryValue> </ServiceOptionsCharges> <TotalCharges> <CurrencyCode>USD</CurrencyCode> <MonetaryValue>52.16</MonetaryValue> </TotalCharges> <Weight>10.0</Weight> <BillingWeight> <UnitOfMeasurement> <Code>LBS</Code> </UnitOfMeasurement> <Weight>10.0</Weight> </BillingWeight> </RatedPackage> </RatedShipment> </RatingServiceSelectionResponse>
Any feedback would be appreciated
Thank you!
-
Thank you very much Ben!
The fixed worked well. I figured out the rest from there.
Sorry it took so long to respond. I believe I got distracted when I last read your feedback.
-
I have this multidimensional array which I'll name "original":
$original=
array
0 =>
array
'animal' => 'cats'
'quantity' => 1
1 =>
array
'animal' => 'dogs'
'quantity' => '1'
2 =>
array
'animal' => 'cats'
'quantity' => '3'
However, I want to merge internal arrays with the same animal to produce this new array:
$new=
array
0 =>
array
'animal' => 'cats'
'quantity' => 4
1 =>
array
'animal' => 'dogs'
'quantity' => '1'
I've been trying for hours, but can't figure it out.
I've tried the following code, but get "Fatal error: Unsupported operand types" (Referring to line 11).
1 $new = array();
2 foreach($original as $entity)
3 {
4 if(!isset($new[$entity["animal"]]))
5 {
6 $new[$entity["animal"]] = array(
7 "animal" => $entity["animal"],
8 "quantity" => 0,
9 );
10 }
11 $new[$entity["animal"]] += $entity["quantity"];
12 }
So, I don't know what I'm doing and I could really use some help from the experts.
-
I ended up figuring it out.
This works:
if($_FILES['img1']['tmp_name'] == ""){}
-
I am trying to copy a bunch of text which consists of several different paragraphs (with empty space in between them).
Then I would like to paste this text into a form's textarea field.
When I submit the form I would like to clean the information with something like the preg_replace below.
$cleaned_info = preg_replace('#[^A-Za-z0-9)(,\'\.\-!?$" "" "]#i', '', $_POST['info']);
How do I preserve the empty lines between paragraphs (which I'm assuming are hard-returns or whatever)?
Adding \n to the things which I would like to preserve didn't work.
-
I have a form with an input field of type="file" name="img1" id="img1".
When the form is submitted, I would like to make sure the user selected a file before my script continues.
I've tried:
if(!isset($_POST['img1'])){
$message="An image file was not selected.";
}
...but that isn't working.
What am I missing?
-
Thank you Ben! That was what I was looking for! And I read through the information you linked for me.
I have another question now.
Let's say you wanted to SELECT * ,let's say 85 columns, of a database table with our query. From what I'm gathering, (and I could be wrong), I would have to type in 85 different variables to bind the results to.
$stmt->bind_result(85 separate variables HERE);
What a pain in the butt THAT would be...
Isn't there an easier way, so that I could SELECT *, but only assign five of them to work with? I mean you can pick and choose which gathered info to work with in the following example: (yes, I realize that this example is an sql query and not a prepared-mysqli one like I am looking for)
$sql=mysql_query("SELECT * FROM friends WHERE sex = 'F'");
$friendCount = mysql_num_rows($sql);
if ($friendCount > 0) {
while($row = mysql_fetch_array($sql)){
$name = $row["name"];
$age = $row["age"];
$favoriteColor = $row["favoriteColor"];
}
}
And one more question...
How do I get a row count (like the one on line 2 of this example) in the prepared-mysqli version?
-
How are you trying to use it? Are you getting any specific error messages?
I don't really get any specific issues with your sample code. For example, this works the way I'd expect:
<?php $name = 'test'; $list = "<a href='editStuff.php?name=$name'>Click Here</a>"; echo $list;
Yes, but the example you have shown assigns the variable $name with 'test'. You need to store a variable that contains an apostrophe to see what I mean. Such as $name = "Johnny's".
-
So, let's say you have to work with a variable that has a single quote in it like:
$name = "Johnny's Thing";
I figured out how to work with it in mysql by using mysql_real_escape_string(). But how do I work with it in this instance?:
$list .= "<a href='editStuff.php?name=$name'>Click Here</a>";
The single quote inside the variable is not allowing it to work correctly.
I've tried addslashes(), but that doesn't seem to help.
Any suggestions?
-
I tried using the examples you provided, but those examples were not prepared statements. My new try at the mysqlI statment looks like this:
$sex="F";
if ($result = $mysqli->prepare("SELECT * FROM friends WHERE sex = ?")){
$result->bind_param("s", $sex);
$result->execute();
$result->store_result();
if ($result->num_rows != 0){
while ($row = $result->fetch_object()){
$name = $row->name;
$age = $row->age;
$favoriteColor = $row->favoriteColor;
$list .= "Here is the result->num_rows: ".$result->num_rows."<br />Here is name: ".$name."<br />Here is age: ".$age."<br />Here is $favoriteColor: ".$favoriteColor."<br /><br />";
}
}
$statement->close();
}
I'm now getting this error referring to the while loop:
Fatal error: Call to undefined method mysqli_stmt::fetch_object()
I just need the mysqli code to do EXACTLY the same thing that the mysql code does (in my initial example).
-
Warning: mysqli_fetch_array() expects parameter 1 to be mysqli_result, object given
This is referring to the if-statement area (of the mysqlI version)
-
//I would like to convert this mysql code to a mysqlI version:
$sql=mysql_query("SELECT * FROM friends WHERE sex = 'F'");
$friendCount = mysql_num_rows($sql);
if ($friendCount > 0) {
while($row = mysql_fetch_array($sql)){
$name = $row["name"];
$age = $row["age"];
$favoriteColor = $row["favoriteColor"];
$list1 .= "Here is the name: ".$name."<br />Here is age: ".$age."<br />Here is the favorite color: ".$favoriteColor."<br /><br />";
}
}
//Here is my shot at the mysqlI version:
$sex="F";
$statement = $mysqli->prepare("SELECT * FROM friends WHERE sex = ?");
$statement->bind_param("s", $sex);
$statement->execute();
$statement->store_result();
if ($statement->num_rows != 0){
while($row = mysqli_fetch_array($mysqli)){
$name = $row["name"];
$age = $row["age"];
$favoriteColor = $row["favoriteColor"];
$list .= "Here is the name: ".$name."<br />Here is age: ".$age."<br />Here is the favorite color: ".$favoriteColor."<br /><br />";
}
}
$statement->close();
I'm pretty sure my problem is in the if-statement area (of the mysqlI version), but I have no idea what to put there. Can someone help me out please?
Occasional Php Warning When Looping Multiple Mysqli Queries
in General Programming
Posted
My PHP script for mysqli queries works most of the time, but while running a loop that queries database many times, occasionally I'll get Warning:
Can someone tell me if I need to modify the structure of my queries to make it work better? Here's my example:
<?php
$host = "localhost";
$username = "blah_blah";
$password = "blah_blah";
$database = "blah_blah";
$mysqli = new mysqli($host, $username, $password, $database,0,'/var/lib/mysql/mysql.sock');
$mysqli->set_charset('utf8');
if ($stmt = $mysqli->prepare("SELECT column_A FROM table_X WHERE column_B=? AND column_C=? LIMIT 1")){
$stmt->bind_param("si", $value_1, $value_2);
$stmt->execute();
$stmt->bind_result($column_A_value);
$stmt->fetch();
$stmt->close();
}
?>