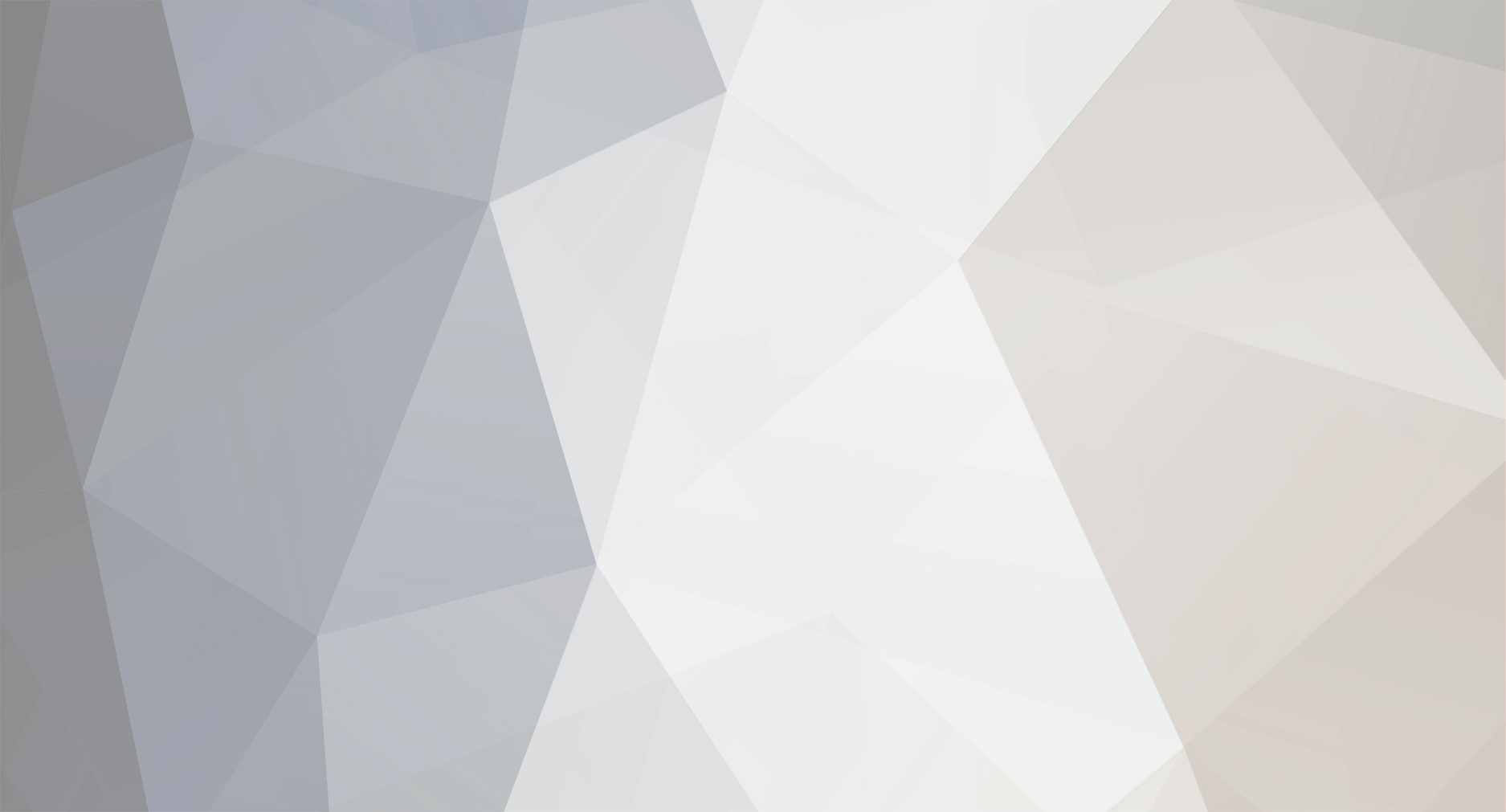
jsmith1981
-
Posts
38 -
Joined
-
Last visited
Content Type
Profiles
Forums
Events
Downloads
Gallery
Store
Posts posted by jsmith1981
-
-
Yea I did that and sadly they're both comparing so would return to be true, so there's nothing wrong within itself just it's not behaving the way I'd want it to.
I actually played around with another example another developer was using and came up with this that works:
case 'add': // we need all the details of the product so we can add it to the cart (the needed data that means) $sql = sprintf("SELECT id, name, price FROM products WHERE id = '%d'", $prodid); // query the products table: $result = mysql_query($sql); // if no result send the user back the the home page: if(!$result) { header("Location: index.php"); // else construct the ability to add the product to the cart, if the result is true and counts for 1 row: } else if ($result && mysql_num_rows($result) > 0) { // get the one row: $row = mysql_fetch_row($result); $tmp_product = array('id'=>$row[0], 'name'=>$row[1], 'price'=>$row[2], 'qty'=>$_POST['qty']); } $cart_count = count($_SESSION['cart']); if($cart_count === 0) { $_SESSION['cart'][] = $tmp_product; // this is fine! } else { $already_in_cart = false; for($i=0; $i<$cart_count; $i++) { // loop through the array rows: // if the id of the product already is in there then add to it: if($_SESSION['cart'][$i]['id'] === $tmp_product['id']) { $_SESSION['cart'][$i]['qty'] = $_SESSION['cart'][$i]['qty'] + $tmp_product['qty']; $already_in_cart = true; break; } } if($already_in_cart === false) { $_SESSION['cart'][] = $tmp_product; } } // end of adding product break;
Thank you so much for your help Ben.
-
// we need all the details of the product so we can add it to the cart (the needed data that means) $sql = sprintf("SELECT id, name, price FROM products WHERE id = '%d'", $prodid); // query the products table: $result = mysql_query($sql); // if no result send the user back the the home page: if(!$result) { header("Location: index.php"); // else construct the ability to add the product to the cart, if the result is true and counts for 1 row: } else if ($result && mysql_num_rows($result) == 1) { // get the one row: $row = mysql_fetch_row($result); $tmp_product = array('id'=>$row[0], 'name'=>$row[1], 'price'=>$row[2], 'qty'=>$_POST['qty']); } $cart_count = count($_SESSION['cart']); if($cart_count == 0) { $_SESSION['cart'][] = $tmp_product; } else if($cart_count > 0) { // if the array has anything in it: for($i=0; $i<$cart_count; $i++) { // loop through the array rows: // if the id of the product already is in there then add to it: if($tmp_product['id'] == $_SESSION['cart'][$i]['id']) { // adds to the already there qty: preincrement actually I think $_SESSION['cart'][$i]['qty'] += $tmp_product['qty']; } else if ($tmp_product['id'] != $_SESSION['cart'][$i]['id']) { // otherwise add to it regardless of the conditional kind of... $_SESSION['cart'][] = $tmp_product; } } } // end of adding product
That's the whole process for the add to cart part of the code I wrote, it's really kind of half going off what I can remember (or lack of lol) what's in 2 different books as a kind of inspiration.
I appreciate your next reply,
Jeremy.
-
I have some weird problem here,
The code is:
if($cart_count == 0) { $_SESSION['cart'][] = $tmp_product; } else if($cart_count > 0) { // if the array has anything in it: for($i=0; $i<$cart_count; $i++) { // loop through the array rows: // if the id of the product already is in there then add to it: if($_SESSION['cart'][$i]['id'] === $tmp_product['id']) { // adds to the already there qty: preincrement actually I think $_SESSION['cart'][$i]['qty'] += $tmp_product['qty']; } else { // otherwise add to it regardless of the conditional kind of... $_SESSION['cart'][] = $tmp_product; } } }
The problem I am having is when I add the same product again it updates the qty that's fine works perfectly but if I was to go and add another product say product 2 as opposed to product 1 (that was added just prior) it keeps adding rows to the array, what's wrong with my logic to suggest it does this?
It must be something pretty obvious I am missing I just can't see it.
I appreciate anyone's help,
Jeremy.
-
In a nut shell really all a proxy is (whether its a web site or server) is something that does some action on behalf of another.
Put it simply you ask the proxy to do something (something it was obviously designed to do) and it will do that for you, then send you back that information without leaving any trace that it was you that wanted that.
That's all proxy's are.
Though to answer your question usually yes that kind of thing would work, but in reality it could be (I would have thought of the way you're describing the situation) it'd be more to do with a geographic aspect of your searching, it'd not be because of blocking really I wouldn't have thought.
What proxy's are you using by chance?
-
If I don't want to accept that cookies, then what happens? Means I don't want to store cookies to my pc, then what should I do to remove it?
To answer your first one, some services on a site won't be available, like you won't be able to login for example (that's probably the biggest one), minor features of a site shouldn't be a problem but that's a big one being able to login for some sites, or purchasing items they usually (least in my experience in ecommerce web development) won't allow you to add things to a basket. Though having said that some do use a database structure and save the contents of your basket to their server (but you must have loads of data free to be able to do that if you're running a shop with allot of customers).
To remove it would be determined by what browser you're using, clearing out the cookies simply would work.
Just a heads up on security I have seen time and time again on the web (this isn't meant to be a horrible comment and I've had very little sleep), though storing a userid in a cookie is a pretty bad idea if say you're using a cms like wordpress (if you aren't then it's not as bad but still you'd be best off not storing that kind of info in a cookie) because hackers (if you have say wp_ at the start of every table name) would be able to try and at least hack into your database and gain user login information a really bad thing if you're running any kind of site dealing with users details.
-
In a nutshell a management KVM/Qemu high end Virtualization, VirtualBox (have good old Windows 3.11 running, wanted to have some fun with QBASIC the first ever programming language I learnt to program in, had some old sessions work I used to do as a student, proper nostalgia, yes I would have to admit I am a real self confessed nerd
) and then my 2 live VPS's using KVM/Qemu.
I run the local management KVM/Qemu in graphical mode on a host operating system with X Windows Server (gnome), basically because I couldn't seem to get the text version working like I used to be able to do, weird as I ran through exactly what I tried doing years ago, oh well works for my needs so....
-
Hmm not sure why this wasn't working but have got it now.
-
I have a very basic (as simple as you can possibly get) ajax script.
Problem is never seems to work when I type in, but when I compare line by line with the most basic example on the w3schools (again that works when I copy and paste, but I hate doing that, despise it personally with myself).
Works when I copied and pasted, then compared line by line mine to theirs, but it's just still not working makes no sense to me at all.
Could someone help me with this?
function loadXMLDoc() { var xmlhttp; if(window.XMLHttpRequest) { xmlhttp=new XMLHttpRequest(); } else { xmlhttp=new ActiveXObject("Microsoft.XMLHTTP"); } xmlhttp.onreadystatechange=function() { alert(xmlhttp); } }
It's the w3schools one: http://www.w3schools.com/ajax/tryit.asp?filename=tryajax_first see when I copy up to where I have the alert, I change the w3schools example and it works, just baffling me to no end, what's wrong with what I am doing?
I'd really love to know.
Thanks in advance everyone
Jeremy.
-
In fact no forget what I said it's working lol, going to get this into some kind of order then rock on with it.
Playing around with theory's fun
-
Oh no probs Andrea, I think I have this understood now, I think it's just the V part of MVC that's causing the problem.
I thought why not just look into where you go when you look at the site, like home and whatever (index.php) is the kind of page it goes to (gets a bit confusing at times) but if I set it to go to the home page I have made in my logic I think it'll be fine.
Because when I go to look at any of the products and using the breadcrumb plugin it goes back to the GET part of the address and finds the root category for products and displays them all, I might just put in some statically done previews (like the previous showsite I showed you and you very kindly offered some advice on).
Thanks ever so much for your reply, though if anyone else who knows more about php wants to advise me on this that'd be wonderful
Take care Andrea,
Jeremy.
(as an edit to this post, least my theme's robust haha, tried it on a test sandbox I have a real fully working hardware VPS server and that works, it's like I wanted to kind of jump up and down and go yea lol, very sad behaviour haha
)
-
Ok sorry I fixed the end of file problem, using this logic:
<?php /* Template Name: Homepage */ if ( ! defined( 'ABSPATH' ) ) exit; // Exit if accessed directly // get_header(); // this is now deactivated! get_header('shop'); do_action('woocommerce_before_main_content'); ?> <?php if ( apply_filters( 'woocommerce_show_page_title', true ) ) : ?> <h1 class="page-title"><?php woocommerce_page_title(); ?></h1><!-- page heading basically --> <?php endif; ?> <?php // do_action( 'woocommerce_archive_description' ); // keep this disabled for now ?> <div id="products-banner"> <?php if ( have_posts() ) : ?> <?php do_action( 'woocommerce_before_shop_loop' ); ?> <?php woocommerce_product_loop_start(); ?> <?php woocommerce_product_subcategories(); ?> <?php while ( have_posts() ) : the_post(); ?> <?php woocommerce_get_template_part( 'content', 'product' ); ?> <?php endwhile; // end of the loop. ?> <?php woocommerce_product_loop_end(); ?> <?php do_action( 'woocommerce_after_shop_loop' ); ?> <?php elseif ( ! woocommerce_product_subcategories( array( 'before' => woocommerce_product_loop_start( false ), 'after' => woocommerce_product_loop_end( false ) ) ) ) : ?> <?php woocommerce_get_template( 'loop/no-products-found.php' ); ?> <?php endif; ?> <?php do_action('woocommerce_after_main_content'); ?> </div> <div class="footer"> <ul> <li>Link 1</li> </ul> </div> <?php get_footer(); ?>
I have a random generated test product with whatever details (not that any of them should stop it from showing), but I put the above code in say home.php
But literally nothing shows up, I will rerun through my settings but does anyone have a clue if it's the logic above that's stopping them from showing? (or it rather depending on if you've got one or whatever number of products lol).
Doing really well with my logical assumptions though, actually this is a heck of allot easier than I first thought, just this is getting in the way. Interesting though.
Look forward hearing anyone's help,
Jeremy.
-
Having problems integrating woocommerce.
I am working on showing just a list of products to begin with, I mean taking the contents of 'archive-product.php' from the templates directory in the woocommerce plugin folder (don't need to explain where that is).
I have got the following:
<?php /* Template Name: Homepage */ if ( ! defined( 'ABSPATH' ) ) exit; // Exit if accessed directly // get_header(); // this is now deactivated! get_header('shop'); /** * woocommerce_before_main_content hook * * @hooked woocommerce_output_content_wrapper - 10 (outputs opening divs for the content) * @hooked woocommerce_breadcrumb - 20 */ do_action('woocommerce_before_main_content'); ?> <?php if ( apply_filters( 'woocommerce_show_page_title', true ) ) : ?> <h1 class="page-title"><?php woocommerce_page_title(); ?></h1><!-- page heading basically --> <?php endif; ?> <?php // do_action( 'woocommerce_archive_description' ); // keep this disabled for now ?> <div id="products-banner"> <?php if ( have_posts() ) : ?> <?php /** * woocommerce_before_shop_loop hook * * @hooked woocommerce_result_count - 20 * @hooked woocommerce_catalog_ordering - 30 */ do_action( 'woocommerce_before_shop_loop' ); ?> <?php woocommerce_product_loop_start(); ?> <?php woocommerce_product_subcategories(); ?> <?php while ( have_posts() ) : the_post(); ?> <?php woocommerce_get_template_part( 'content', 'product' ); ?> <?php endwhile; // end of the loop. ?> <?php woocommerce_product_loop_end(); ?> <?php /** * woocommerce_after_shop_loop hook * * @hooked woocommerce_pagination - 10 */ do_action( 'woocommerce_after_shop_loop' ); ?> </div> <div class="footer"> <ul> <li>Link 1</li> </ul> </div> <?php get_footer(); ?>
It's then coming up with (when I refresh my browser) with the following:
'Parse error: syntax error, unexpected end of file' then shows the file I am editing which is basically home.php (my wordpress template file) on the last line, I really am confused by this.
Is there anything I am doing that's completely wrong here? I mean i went through judging by the logic I would have thought this should work?
Any help's massively appreciated,
Jeremy
PS Sorry to edit this but I shortened it to this:
<?php /* Template Name: Homepage */ if ( ! defined( 'ABSPATH' ) ) exit; // Exit if accessed directly // get_header(); // this is now deactivated! get_header('shop'); do_action('woocommerce_before_main_content'); ?> <?php if ( apply_filters( 'woocommerce_show_page_title', true ) ) : ?> <h1 class="page-title"><?php woocommerce_page_title(); ?></h1><!-- page heading basically --> <?php endif; ?> <?php // do_action( 'woocommerce_archive_description' ); // keep this disabled for now ?> <div id="products-banner"> <?php if ( have_posts() ) : ?> <?php do_action( 'woocommerce_before_shop_loop' ); ?> <?php woocommerce_product_loop_start(); ?> <?php woocommerce_product_subcategories(); ?> <?php while ( have_posts() ) : the_post(); ?> <?php woocommerce_get_template_part( 'content', 'product' ); ?> <?php endwhile; // end of the loop. ?> <?php woocommerce_product_loop_end(); ?> <?php do_action( 'woocommerce_after_shop_loop' ); ?> </div> <div class="footer"> <ul> <li>Link 1</li> </ul> </div> <?php get_footer(); ?>
Just basically because I know what everything is, most of what wp wants has to be in the top part of the file (source) have I missed something off like an actual product file or something perhaps? I am really just clueless as to what's going wrong.
-
I'll just list what I notice one by one:
- The red of Login and Register on the black background is bad - not enough contrast
- I kind of get the "Sweet Sparkle Hampers", but no idea what the 'Wedding Hire" is supposed to mean.
- Your footer text is cut off - I only see as far as CMS Provided t (maybe that's a chopped of b --- that's it.
- There is way too much pink for me - but that's personal preference.
Looking at the code:
- Hidden comments can be useful, I think you may be going a bit overboard. meta tags are obvious, so is title start and end, and IMO does not require any more explanation than the tags themselves. However, this is personal preference, and commenting on everything is not wrong.
- You are starting your footer with a div class footer but are closing it with the HMTL5 closing footer tag - pick one.
The banner (static element) I was having problems adding the banner further up, so thats fixed now, I was thinking about the problems with yea the text at the top, not enough contrast
I will go through it with the person I am doing it for about yea the hiring part. It's more they are like an agent going between hiring companies and offering that. But I shall confirm, it's just on her facebook page I thought about keeping it the same as that but ideally I need to confirm.
Thank you ever so much
PS with regards to the comments I shall clear it up but it's just so I can remember where everything is, once it goes into production/live status then I will remove allot of them
Really it's just an exercise for me to understand how wordpress theme's are developed for me personally.
-
I am actually thinking of redoing (well not thinking will redo it at some point) but pondering using the same thing.
A mentor of mine (who set up pitchspring for people to pitch new businesses and connect them with possible investors), used a similar thing in that you have say 3 things you do when going to that site. You're either a entrepreneur, an investor or (I actually can't remember the other) and it's just those options, taking you to a consultation contact page to book a call back with the investors if you're the earlier person/user.
Quite cool stuff if you ask me personally (think he referred to it as a cause for action or something like that).
-
Love this one "There's a golden Jesus thing." actually made me chuckle. It is rubbish though, shaking my head at the rest on the 2012, or whatever list.
I have seen some I'm going to improve for places around here, geographically speaking of course, it's clear to me some of them (ok most of them), they've taken templates and tried to modify them without checking browser (or even knowing about) compatibility those make me weep more.
Finally great post!
-
Hello hope you're well?
I found this (personally speaking of course) a great resource for starting off on your own http://www.siteground.com/tutorials/wordpress/wordpress_create_theme.htm
Then just take things out of a theme you like the look of, get it down to its basic HTML (what I found good was this function
<?php remove_filter ('the_content', 'wpautop'); ?>
Every time you make any post it annoyingly puts in the p tags in every post (no matter what markup is in the post content, either side of your content it puts in yea the p tags. The above code removes them.
Hope this helps?
-
I just wondered what people think of this site here (have been working on this exclusively): www.sweetsparklehampers.com
As you can tell I haven't finished the grey bar (will eventually, like allot (ok all) of the menu's completely dynamic yet), but what's your honest opinions of it, I'd really like to hear them.
Also what's your best advice of theme development with regards to woocommerce? I am not sure about how to integrate this (when I've finished the front page layout of the site). I want to make a good job of this since this is the first portfolio site I have done ever really.
I appreciate your advice in advance,
Jeremy
-
Basically this is what I have now and it works:
.footer ul li { list-style-type:none; }
Thanks ever so much again, your help's been amazing!
Jeremy.
-
well DUH (this is for me, not you) --- bullets are removed via list-style-type: none. The text-docoration: none is for the underline.
No no you're quite alright haha thank you sooo much for your help, I can't tell you how much I appreciate that response.
I thought I put that in further up, funny eh? Oh well I will defo try that, I thought it'd make more sense if I just copied in a screendump of what I was having problems with because I clearly confused myself haha with yea what you mentioned before haha.
Thanks ever so much again, I am going to definitely post your great reply to my private servers blog (one that's not available on the Internet as all the ports are blocked), you're great thank you thank you thank you.
All the best,
Jeremy.
-
I am not a noob when it comes to say sys admin (dont tons of work in that respect, so that's my background), personally I find the tips in the book CSS Mastery by Andy Budd full of amazing tips part of that and yea what the OP said once you break things down and really experiment (by seeing what happens to an element when I do this..) in exactly the same way when I came to fully understand Regex's (I would strongly recommend noob's going into any kind of programming that has functions for regex's not to start off doing them they're very cryptic) but yea I experimented now I can (when I think about it hard) even diagnose regex's or explain what they do by just looking at them closely.
It's the same approach I have to CSS.
One thing I'd say not even that sometimes with what the OP (absolutely no offence meant by this, this is purely just my opinion), if all you have for say a top heading (H1 if you will) and its just purely that nothing else why bother putting that (if it's on its own) as an ID? Unless you wanted a similar style to that on other parts of the page, but if not just use the type/element selectors!
-
Just a point out really, which mode of editing are you using? There's visual and text, using text this won't happen. You'd have to enter all the html yourself
-
I don't understand why I can't remove the bullet styling of the list still, tried again (I tried changing both the element and selectors to a class, thus my silly mistake in my first post in this thread, changed the CSS but not the markup), does anyone have an idea as to why?
-
using the html5 way of coding and using <footer> vs. the former <div class(or id)="footer"> your CSS needs to address just footer -- not .(for class which you don't have)footer --- in plain - No Period in front of the footer.
I did already do that, must have got confused with editing (got allot of things on at the moment), but it's still not working even when I address that problem (why it worked in compat mode in IE10).
Please see attached.
-
I am having problems getting my head around what I have done here to cause this, but basically I want say a list of links to appear at the bottom of my site (above a static element at the bottom) but there's a snag, no matter how hard I try can't remove the bullet point from even one list (li) element. When I try with this CSS logic:
/* Theme Name: Sweet Sparkle Hampers Author: Jeremy Smith */ * { padding: 0; margin: 0; } body { font-family:Verdana, Geneva, sans-serif; color: #000; background: #f784a5; } h1 { font-size: 1.8em; color: #f784a5; } h2 { font-size: 1em; } a { text-decoration:none; } #banner-menu { display: block; position: fixed; width: 100%; z-index: 999; background: #000; padding: 5px 0px 5px 0px; font-size: 0.9em; } #banner-menu .user-nav { margin-right: 20px; } #banner-menu .user-nav li { list-style: none; padding: 0 15px 0 0; display:inline; float:right; } #banner-menu .user-nav li a { color: white; } #banner-menu .user-nav .user-register { float: left; color: red; padding-left: 20px; } #banner-menu .user-nav .user-register a { color: red; } #page { background: #fff; position:relative; top: 40px; /* margin: 0 60px 0 60px; */ margin: auto; width: 970px; height: 680px; padding: 25px 25px 15px 25px; /* REMEMBER ITS: */ /* border: 1px solid #000; /* used for guidance only! */ } #heading { display:inline; } #heading h1 { text-transform: uppercase; padding-bottom: 5px; } #heading h2 { padding-bottom: 45px; } #products-banner { height: 400px; text-align:center; margin-bottom: 70px; } #products-banner img { /* try to remove padding within images but not really to worry */ padding: 0; margin: 0; } h3#home-message { text-align:center; color: red; text-transform: uppercase; padding-top: 20px; padding-bottom: 20px; } h3#home-message a:visited { /* NOTHING AS YET */ color: red; } h3#home-message a:hover { /* NOTHING AS YET just the same colour */ color: red; } .footer { /* nothing as yet */ background: #cccccc; width: 910px; margin: auto; padding-top: 20px; padding-bottom: 20px; } .footer ul li { text-decoration: none; } /* now for the footer: */ #site-footer { display: block; position: fixed; width: 100%; z-index: 999; background: #000; padding: 5px 0px 5px 0px; font-size: 0.9em; bottom: 0; } #site-footer p#author { color: white; float: right; padding-right: 35px; } #site-footer p#author a { color: white; text-decoration: none; } #site-footer p#author a:link { color: white; text-decoration: none; } #site-footer p#author a:hover { color: white; text-decoration: none; } #site-footer p#author a:visited { color: white; text-decoration: none; }
(sorry it is rather a long one)
Then with this markup:
<!DOCTYPE html> <html lang="en-US"> <head> <!-- META TAGS --> <meta charset="UTF-8" /> <!-- END META TAGS --> <!-- TITLE START --> <title>Sweet Sparkle Hampers & Wedding Hire | Sweet Sparkle Hampers makes gift hampers for all occasions</title> <!-- TITLE END --> <link rel="stylesheet" href="http://www.sweetsparklehampers.com/wp-content/themes/sweetsparkle/style.css"> <!--[if lt IE 9]> <script src="http://www.sweetsparklehampers.com/wp-content/themes/sweetsparkle/js/htmlshiv.js"></script> <![endif]--> <meta name="description" content="Sweet Sparkle Hampers make gift hampers for all occasions from birthdays to new baby there is something for everyone to suit all budgets. We also hire out table centre pieces for your special day as well as favours"> <meta name="keywords" content="hamper gifts, hampers, all occasions birthdays to new baby babies, hiring centre pieces, special day"> <meta name="author" content="Jeremy Smith | Web Services Consultancy, Harrogate, UK (jeremysmith.me.uk)"> </head> <body> <!-- STATIC TOP MENU FOR USER --> <div id="banner-menu"> <ul class="user-nav"> <li><a href="#">Checkout</a></li> <li><a href="#">Basket</a></li> <li><a href="#">Wishlist</a></li> <li><a href="#">My Account</a></li> <li class="user-register"><a href="#">Login|Register</a></li> </ul> </div> <!-- END OF STATIC TOP MENU FOR USER --> <div id="page"> <!-- PAGE TITLE & DESCRIPTION --> <div id="heading"> <h1>Sweet Sparkle Hampers & Wedding Hire</h1> <h2>Sweet Sparkle Hampers makes gift hampers for all occasions</h2> </div> <!-- END PAGE TITLE & DESCRIPTION --> <div id="products-banner"> <img src="http://www.sweetsparklehampers.com/wp-content/uploads/2013/11/1004544_642654019088856_612530407_n-banner.jpg" alt=""> <img src="http://www.sweetsparklehampers.com/wp-content/uploads/2013/11/998315_639162619437996_1265361729_n-banner.jpg" alt=""> <img src="http://www.sweetsparklehampers.com/wp-content/uploads/2013/11/482414_642649705755954_1533956264_n-banner.jpg" alt=""> <h3 id="home-message" title="Shop Coming Soon"><a href="">Shop Coming Soon</a></h3> </div> <footer> <ul> <li>Link 1</li> </ul> </footer> </div> <!-- author info banner --> <div id="site-footer"> <p id="author">Design & Theme (Sweet Sparkle) by <a href="http://jeremysmith.me.uk/">Jeremy Smith</a> | CMS Provided by <a href="http://wordpress.org/" target="_blank">wordpress.org</a></p> </div> <!-- end author info banner --> <!-- END OF PAGE --> </body> </html>
The bullet point just stays there it's this point that I mean:
<div class="footer"> <ul> <li>Link 1</li> </ul> </div>
With this added CSS:
.footer { /* nothing as yet */ background: #cccccc; width: 910px; margin: auto; padding-top: 20px; padding-bottom: 20px; } .footer ul li { text-decoration: none; }
Though when I turn Compat mode on in IE10 it works, not sure what I have done wrong here, there's obviously a conflict. Any help's massively appreciated.
Thanks,
Jeremy.
Continually Updating A HTML Element In Vanilla JavaScript
in Javascript
Posted · Edited by jsmith1981
Didnt put the language for the code
Doing some great work to finally understand how to insert data into a database using JavaScript AJAX and PHP
But the problem I'm having is this, when inserting say a post (a basic commenting system is what I am doing just for a kind of proof of concept sort of thing so I can understand whats going on as such) it inserts the post but needing to refresh the page to see the new comment/post/data
Is there any better way of say doing this like so when inserting it automatically appears in the feed, have this essentially right now:
Essentially all the responseText is the results of all data from the database in a nutshell nothing complicated, a kind of guestbook of sorts, tried putting it in a while loop thats infinite but that keeps crippling the tab in the browser or nothing appears
Like I was thinking of doing it so that yeah just updates once until a post is made to the database, but then thought would it be possible to make it sort of live updating
Any helps much appreciated
Jeremy.