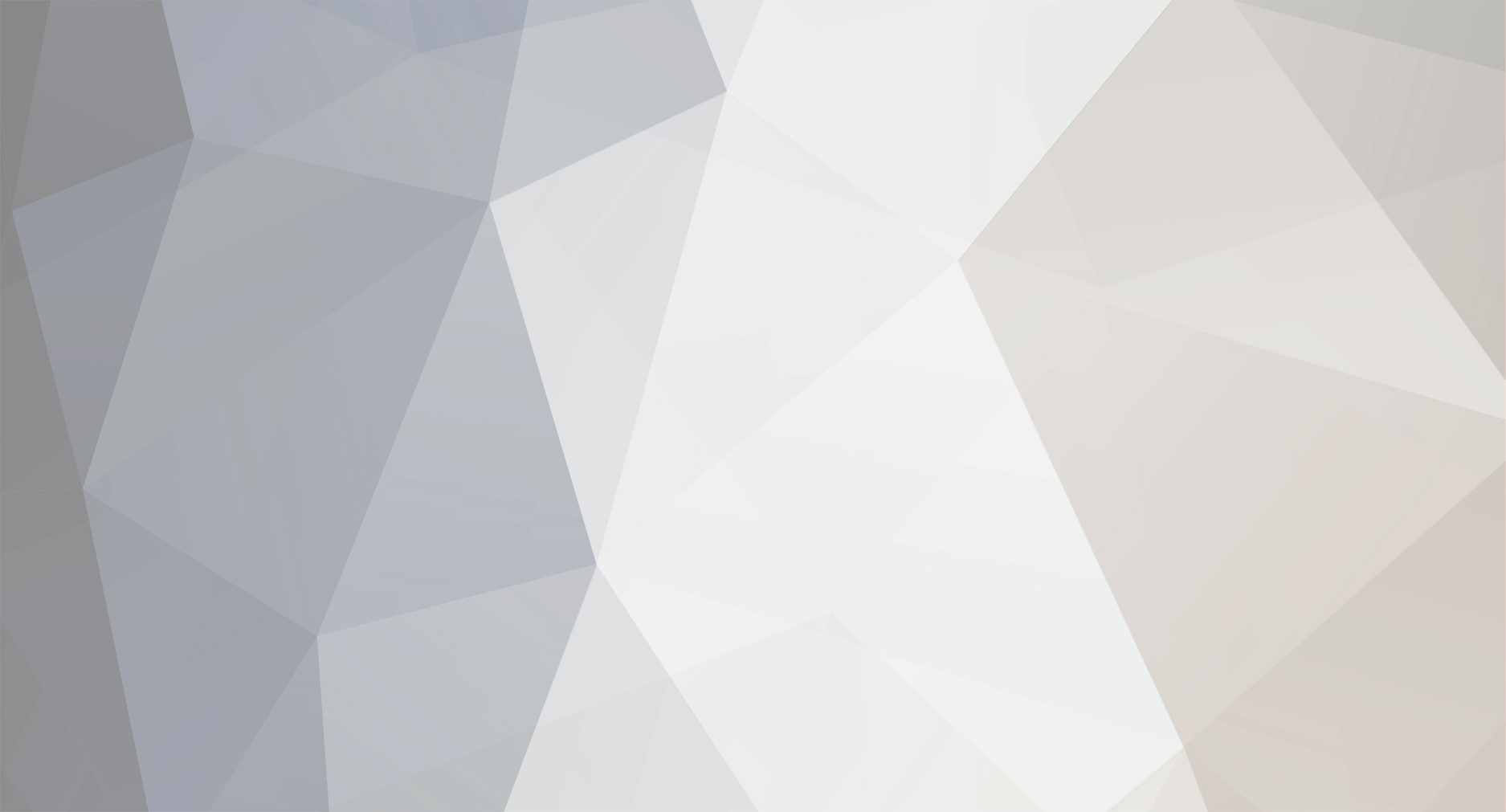
dhahlen
-
Posts
20 -
Joined
-
Last visited
Content Type
Profiles
Forums
Events
Downloads
Gallery
Store
Posts posted by dhahlen
-
-
I am now working with Exceptions.
The goal here is to throw a catch/try block in 3 different sections.
Here is an example provided:
//A function that throws an exception, for the example only function TryThis() { throw new Exception("I tried it, but it didn't work"); } try { TryThis(); } catch(Exception $e) { echo "An error occured with the following error<br />" . $e->getMessage(); } Prints An error occured with the following error I tried it, but it didn't work
A couple of questions:
Question 1: Can you use regex inside of OOP? (lets say I want to validate the format of an name or e-mail address. If it's not in the correct format, then throw an exception, then display a message with the catch) I already have regular expressions in place to validate the e-mail, but I'd like to have it thrown an exception instead. Here is the code I use to validate e-mail:
<?php $stringSubject = $_POST['txtSubject']; $stringSubject = str_ireplace("Estrella Mountain","EMCC",$stringSubject); $stringMessage = $_POST['txtMsg']; $stringMessage = str_ireplace("Estrella Mountain","EMCC",$stringMessage); $validemail = $_POST['txtEmail']; $myemailpattern = '^[a-zA-Z0-9\.-_]{1,}@[a-zA-Z0-9\.-_]{1,}(.com|.net|.edu){1}'; if(eregi($myemailpattern,$validemail)) { if(isset($_POST['txtEmail']) && isset($_POST['txtSubject']) && isset($_POST['txtMsg'])) { $from = "From: " . $_POST['txtEmail'] . "\r\n"; $subject = $stringSubject; $msg = $stringMessage; $result = mail('djwampus@gmail.com',$subject,$msg,$from); if($result) { echo "Your email was successfully sent with the following information<br />"; echo "Email Address: " . $from . "<br />"; echo "Subject: " . $subject . "<br />"; echo "Message: " . $msg . "<br />"; } else { echo "<h1 style=\"color:red;\">An error occured sending the email!</h1>"; } } else { echo "<h1 style=\"color:red;\">All fields must be filled out!</h1>"; } } else { echo "<h1 style=\"color:red;\">Invalid E-mail Address</h1>"; } ?>
Question 2: in the example provided below, they use $value >=0 && $value <=23) - is there anyway to change it so it uses characters versus numbers? For example ($value = '^[a-zA-Z0-9\.-_]{1,}')
Thanks
-
logout.php
<?php session_destroy(); // then use the header function to redirect the user header("location: module8.php"); ?>
form.php
<html> <?php // added include_once("login_class.php"); // added if (isset($_SESSION['loggedin'])) // added { header("location: loggedin.php"); // added } ?> <head> <style type="text/css"> /* Style Code referenced from http://www.webreference.com/programming/css_frames/index.html */ /* Below are the CSS styles which are referenced throughout the webpage */ body { margin:0; border:0; pading:0; height:100%; background:#eee; font-family:arial, verdana, sans-serif; font-size:76%; overflow: hidden; } #header { position:absolute; top:0; left:0; width:100%; height:100px; overflow:auto; text-align:center; background:#53829d; color:#fff; } #footer { position:absolute; bottom:0; left:0; width:100%; height:50px; overflow:auto; text-align:center; /* Aligns the footer text to the center */ background:#73a2bd; } #contents { position:fixed; top:100px; /* This allows the contents of the body to miss the header position */ left:0; bottom:50px; /* This allows the contents of the body to miss the footer position */ right:0; overflow:auto; /* Adds scroll bars if needed */ background:#fff; } /* navlist referenced from http://css.maxdesign.com.au/listamatic/horizontal01.htm */ #navlist li { display: inline; list-style-type: none; padding-right: 20px; } /* Defines the width of the paragraphs */ p {width:500px;} /* for internet explorer */ * html body { padding:120px 0 50px 0; } * html #contents { height:100%; width:100%; } </style> </head> <body> <div id="header"> <?php echo "<h1>Welcome to Darren's Website</h1>";?> <?php echo "<h2>This line uses h2 style with php</h2>";?> </div> <div id="footer"> <h3>Test Footer using h3, aligned center</h3> </div> <div id="contents"> <div id="navcontainer"> <ul id="navlist"> <li id="active"><a href="http://cis166.estrellamountain.edu/DARUP97011/module8.php" id="current">Home</a></li> <li><a href="guestbook.php">Guest Book</a></li> <li><a href="guestbookwrites.php">Guest Book Writes</a></li> <li><a href="mailto:DARUP97011@maricopa.edu">Contact Me</a></li> <li><a href="contents.txt">Page Code</a></li> <li><a href="mailform.php">E-mail Form</a></li> <li><a href="login.php">Login Page</a></li> </ul> </div> <h1>Contents of the Body goes here, using h1 style</h1> <p> Today's Date is <?php echo date("m-d-Y");?> <p> <h2>Login Example</h2> <form action="" method="post"> <!-- leaving the action attribute blank will cause the page to redirect to itself when the submit button is clicked --> <div> <label for="username">Username:</label> <input name="username" id="username" type="text"> <br/> <label for="password">Password:</label> <input name="password" id="password" type="text"> <br/> <input name="submit" value="Login" type="submit"> </div> </form> </body> </html>
login.php
I tried this with and without the
if (isset($_SESSION['loggedin'])) { header("location: module8.php"); }
it yielded the same results. Here is how it sits on the server
<?php session_start(); include_once("login_class.php"); $login = new Login(); if (isset($_SESSION['loggedin'])) { header("location: module8.php"); } if (isset($_POST['submit'])) // this checks if the submit button has been clicked { // if so, process the form $login->processForm(); } else { // if the button hasn't been clicked, show the form $login->generateForm(); } ?> <p> </body> </html>
-
added this
logout.php
<?php session_destroy(); // then use the header function to redirect the user header("location: module8.php"); ?>
When I was clicking on the login page link (form.php) it would keep cycling through the logged in page and the form page (some type of loop, every click would be one or the other, alternating)
I moved the logout page to move me to module8.php, and I included this code above form.php
<?php session_start(); include_once("login_class.php"); $login = new Login(); if (isset($_SESSION['loggedin'])) { header("location: loggedin.php"); } ?>
Now, it seems like the session is not getting destroyed....
-
One other thing to note in case it hasn't been covered in your class... the "!" in "if (!isset($_SESSION['loggedin']))" indicates "NOT". So that line reads, if the session variable is NOT set, do something.
The only time you need to use the checkLogin() function is to make sure that someone who isn't authorized can't access a restricted page. Actually, perhaps a better name for that function would be "checkLoggedIn()" to help reduce confusion.
Ahh, good to know. Removing that ! would redirect a user if they were logged into the session, doing the opposite of what it does currently.
-
Everything appears to be working, which now has me curious on the session_destroy() command
If we go to anywhere on the page, the session is active. If I click the login page again, can I make an option that says "Already Logged in, click here to log out" and then have the log out link perform a session_destroy() ?
This isn't a requirement, but it would be useful in a situation such as this. If someone is logged it and then went to the login page, in most real world scenarios it would tell you the user is already logged in. Also, I understand that we'd normally be authenticating against something like sql or database, versus the method we've completed here.
Thanks for all your help, I'm sure I'll be asking more questions as things progress.
-
Ignore the repetitive css code, I really should create a style page to eliminate all the excess code.
-
login_class.php
<?php class login { var $username = "admin"; var $password = "password"; function __construct() // constructor, PHP5 only { // if you need to do anything when the object is first created, place that code here } function generateForm() { include("form.php"); } function processForm() { if($_POST['username']==$this->username && $_POST['password']==$this->password) { $_SESSION['loggedin'] = TRUE; } else { // show the form using generateForm() function $this->generateForm(); } } function checkLogin($redirect) { // would check if the correct $_SESSION variable is set. If so, user is valid // and can view the requested page. If not, redirect the user using the header() // function to whatever $redirect is set to if (!isset($_SESSION['loggedin'])) { // redirect user header("location: module8.php"); } else { // redirect if user is not logged in header("location: " . $redirect); } } } ?>
login.php
<html> <head> <style type="text/css"> /* Style Code referenced from http://www.webreference.com/programming/css_frames/index.html */ /* Below are the CSS styles which are referenced throughout the webpage */ body { margin:0; border:0; pading:0; height:100%; background:#eee; font-family:arial, verdana, sans-serif; font-size:76%; overflow: hidden; } #header { position:absolute; top:0; left:0; width:100%; height:100px; overflow:auto; text-align:center; background:#53829d; color:#fff; } #footer { position:absolute; bottom:0; left:0; width:100%; height:50px; overflow:auto; text-align:center; /* Aligns the footer text to the center */ background:#73a2bd; } #contents { position:fixed; top:100px; /* This allows the contents of the body to miss the header position */ left:0; bottom:50px; /* This allows the contents of the body to miss the footer position */ right:0; overflow:auto; /* Adds scroll bars if needed */ background:#fff; } /* navlist referenced from http://css.maxdesign.com.au/listamatic/horizontal01.htm */ #navlist li { display: inline; list-style-type: none; padding-right: 20px; } /* Defines the width of the paragraphs */ p {width:500px;} /* for internet explorer */ * html body { padding:120px 0 50px 0; } * html #contents { height:100%; width:100%; } </style> </head> <body> <div id="header"> <?php echo "<h1>Welcome to Darren's Website</h1>";?> <?php echo "<h2>This line uses h2 style with php</h2>";?> </div> <div id="footer"> <h3>Test Footer using h3, aligned center</h3> </div> <div id="contents"> <div id="navcontainer"> <ul id="navlist"> <li id="active"><a href="http://cis166.estrellamountain.edu/DARUP97011/module8.php" id="current">Home</a></li> <li><a href="guestbook.php">Guest Book</a></li> <li><a href="guestbookwrites.php">Guest Book Writes</a></li> <li><a href="mailto:DARUP97011@maricopa.edu">Contact Me</a></li> <li><a href="contents.txt">Page Code</a></li> <li><a href="mailform.php">E-mail Form</a></li> <li><a href="login.php">Login Page</a></li> </ul> </div> <h1>Contents of the Body goes here, using h1 style</h1> <p> Today's Date is <?php echo date("m-d-Y");?> <p> <?php session_start(); include_once("login_class.php"); $login = new Login(); if (isset($_POST['submit'])) // this checks if the submit button has been clicked { // if so, process the form $login->processForm(); } else { // if the button hasn't been clicked, show the form $login->generateForm(); } ?> <p> </body> </html>
loggedin.php
<html> <head> <style type="text/css"> /* Style Code referenced from http://www.webreference.com/programming/css_frames/index.html */ /* Below are the CSS styles which are referenced throughout the webpage */ body { margin:0; border:0; pading:0; height:100%; background:#eee; font-family:arial, verdana, sans-serif; font-size:76%; overflow: hidden; } #header { position:absolute; top:0; left:0; width:100%; height:100px; overflow:auto; text-align:center; background:#53829d; color:#fff; } #footer { position:absolute; bottom:0; left:0; width:100%; height:50px; overflow:auto; text-align:center; /* Aligns the footer text to the center */ background:#73a2bd; } #contents { position:fixed; top:100px; /* This allows the contents of the body to miss the header position */ left:0; bottom:50px; /* This allows the contents of the body to miss the footer position */ right:0; overflow:auto; /* Adds scroll bars if needed */ background:#fff; } /* navlist referenced from http://css.maxdesign.com.au/listamatic/horizontal01.htm */ #navlist li { display: inline; list-style-type: none; padding-right: 20px; } /* Defines the width of the paragraphs */ p {width:500px;} /* for internet explorer */ * html body { padding:120px 0 50px 0; } * html #contents { height:100%; width:100%; } </style> </head> <body> <div id="header"> <?php echo "<h1>Welcome to Darren's Website</h1>";?> <?php echo "<h2>This line uses h2 style with php</h2>";?> </div> <div id="footer"> <h3>Test Footer using h3, aligned center</h3> </div> <div id="contents"> <div id="navcontainer"> <ul id="navlist"> <li id="active"><a href="http://cis166.estrellamountain.edu/DARUP97011/module8.php" id="current">Home</a></li> <li><a href="guestbook.php">Guest Book</a></li> <li><a href="guestbookwrites.php">Guest Book Writes</a></li> <li><a href="mailto:DARUP97011@maricopa.edu">Contact Me</a></li> <li><a href="contents.txt">Page Code</a></li> <li><a href="mailform.php">E-mail Form</a></li> <li><a href="form.php">Login Page</a></li> </ul> </div> <h1>Contents of the Body goes here, using h1 style</h1> <p> Today's Date is <?php echo date("m-d-Y");?> <p> This page uses a combination of html, php, and CSS. All styles are handled by CSS, as well as the navigation menu, header and footer. The page is written in HTML, but is excuted on the server end using php. I have left examples as to what certain header and text formatting look like. The code will also reference pages I have used. I've also provided links for them below. </p> <p> <a href="http://css.maxdesign.com.au/listamatic/horizontal01.htm">Navigation Menu CSS Code referenced here</a> <p> <a href="http://www.webreference.com/programming/css_frames/index.html">CSS Styles referenced here</a> <p> <?php session_start(); include_once("login_class.php"); $login = new Login(); $login->checkLogin('login.php'); ?> You're logged in! </body> </html>
form.php
<html> <head> <style type="text/css"> /* Style Code referenced from http://www.webreference.com/programming/css_frames/index.html */ /* Below are the CSS styles which are referenced throughout the webpage */ body { margin:0; border:0; pading:0; height:100%; background:#eee; font-family:arial, verdana, sans-serif; font-size:76%; overflow: hidden; } #header { position:absolute; top:0; left:0; width:100%; height:100px; overflow:auto; text-align:center; background:#53829d; color:#fff; } #footer { position:absolute; bottom:0; left:0; width:100%; height:50px; overflow:auto; text-align:center; /* Aligns the footer text to the center */ background:#73a2bd; } #contents { position:fixed; top:100px; /* This allows the contents of the body to miss the header position */ left:0; bottom:50px; /* This allows the contents of the body to miss the footer position */ right:0; overflow:auto; /* Adds scroll bars if needed */ background:#fff; } /* navlist referenced from http://css.maxdesign.com.au/listamatic/horizontal01.htm */ #navlist li { display: inline; list-style-type: none; padding-right: 20px; } /* Defines the width of the paragraphs */ p {width:500px;} /* for internet explorer */ * html body { padding:120px 0 50px 0; } * html #contents { height:100%; width:100%; } </style> </head> <body> <div id="header"> <?php echo "<h1>Welcome to Darren's Website</h1>";?> <?php echo "<h2>This line uses h2 style with php</h2>";?> </div> <div id="footer"> <h3>Test Footer using h3, aligned center</h3> </div> <div id="contents"> <div id="navcontainer"> <ul id="navlist"> <li id="active"><a href="http://cis166.estrellamountain.edu/DARUP97011/module8.php" id="current">Home</a></li> <li><a href="guestbook.php">Guest Book</a></li> <li><a href="guestbookwrites.php">Guest Book Writes</a></li> <li><a href="mailto:DARUP97011@maricopa.edu">Contact Me</a></li> <li><a href="contents.txt">Page Code</a></li> <li><a href="mailform.php">E-mail Form</a></li> <li><a href="login.php">Login Page</a></li> </ul> </div> <h1>Contents of the Body goes here, using h1 style</h1> <p> Today's Date is <?php echo date("m-d-Y");?> <p> <h2>Login Example</h2> <form action="" method="post"> <!-- leaving the action attribute blank will cause the page to redirect to itself when the submit button is clicked --> <div> <label for="username">Username:</label> <input name="username" id="username" type="text"> <br/> <label for="password">Password:</label> <input name="password" id="password" type="text"> <br/> <input name="submit" value="Login" type="submit"> </div> </form> </body> </html>
-
Even with the session_start(); located in login.php, loggedin.php, it still does not redirect.
I couldn't simplify it and set the else statement under the processForm to redirect to another page... but for the sake of understanding, I really want to know why it's not working.
How long does the session stay active? I know it's a browser session, so it's temporary.
Thanks for the info on that $redirect, it still confuses me how it checks a file within a file and then that file references another file, this is why I get lost, stuff is going on all over the place.
-
It seems to be getting hung up somewhere, it generates the form if the user/pass is not correct, but it does not redirect.
Also, I do not understand the $redirect syntax
if the user is not logged in, it performs $redirect, but I do not see where this is defined?
If I end up putting the header("location: module8.php") under the $_SESSION['loggedin'] = TRUE, I know it's working.
Something is happening where the checkLogin function is not properly redirecting to the page
-
Well, the login portion works (great!) but it keeps failing when I try to call the generateForm function
I thought you could call a function by it's name.... see below
<?php class login { var $username = "admin"; var $password = "password"; function __construct() // constructor, PHP5 only { // if you need to do anything when the object is first created, place that code here } function generateForm() { include("form.php"); } function processForm() { if($_POST['username']==$this->username && $_POST['password']==$this->password) { $_SESSION['loggedin'] = TRUE; // inserted the header here rather than using isset below // if i remove the header line blow the page will not forward therefore // something else is wrong with the code header("location: " . ' module8.' .'php '); } else { // show the form using generateForm() function // this is also not working generateForm(); } } function checkLogin($redirect) { // would check if the correct $_SESSION variable is set. If so, user is valid // and can view the requested page. If not, redirect the user using the header() // function to whatever $redirect is set to if (!isset($_SESSION['loggedin'])) { // redirect user header("location: " . module8.' .'php '); } else { // Another call to the form which probably does not work generateForm(); } } } ?>
-
<?php class login { var $username = "admin"; var $password = "password"; function __construct() // constructor, PHP5 only { // if you need to do anything when the object is first created, place that code here } function generateForm() { include("form.php"); } function processForm() { if($_POST['username']==$this->username && $_POST['password']==$this->password) { session_start(); $_SESSION['login']="loggedin"; } // set session variable // redirect user using header() function -- see checkLogin() function for example else { // show the form using generateForm() function header("location: " . login.php); } } function checkLogin($redirect) { // would check if the correct $_SESSION variable is set. If so, user is valid // and can view the requested page. If not, redirect the user using the header() // function to whatever $redirect is set to if (!isset($_SESSION['loggedin'])) { // redirect user header("location: " . module8.php); } else { header("location: " . login.php); } } } ?>
-
Maybe my tools are limited.... I'm using textpad for code and the only thing I have to troubleshoot is the browser.
The isset command under checkLogin references a session name called "loggedin"
IN that case, I would have to set my session to generate a session titled "loggedin"
// Starts the Session
session_start();
// Gives the session a name?????
$_SESSION['login']="loggedin";
-
so after the post, we need to do a
session_start(loggedin)
-
Seems like you'd want to do
if($_POST['username']==$this->username && $_POST['password']==$this->password)
No extra "$" after the "->" and you'd want to compare the variables directly, rather than using 'admin' or 'password'.
But this should be referenced in a page other than the login_class.php page?
So far the function processForm() does the following:
-echo's "form processing.."
-verifies the username and password using $this to reference the username/password variable
function processForm()
{
echo "form processing...";
}
{
if($_POST['username']==$this->username && $_POST['password']==$this->password)
}
Now what to do with it afterward, I haven't used session variables. However, I don't think the form process function is complete.
-
No worries. Jumping right in to OOP is a big step if you don't already have a strong understanding of regular procedural PHP, so a bit of confusion is normal. However, being able to understand OOP will definitely help you in the long run.
In addition to the KillerSites tutorials (which it sounds like you've already done) you might want to check out http://net.tutsplus.com/tutorials/php/object-oriented-php-for-beginners/. It's one of the best beginner's articles I know for talking about OOP.
I was reading through that before I posted here
I've updated the page links accordingly. Seems to hang up on form processing...
Makes sense, because the function processForm() doesn't do anything (yet).
Now, when the processForm() function is called, I want to do the following:
if($_POST['username']=='admin' && $_POST['password']=='password')
$this->$username;
$this->$password;
Is this along the right track? I need to review the $this-> material
-
Thanks, yes, I will be working through it slowly. I figured I'd get your templates up and troubleshoot/ask questions as I work through all of them. Be aware that I'll probably get confused several times while attempting to figure all of this out, hah. It really is quite the learning process for me. Things tend to references other pages and bounce around quite a bit, so it's easy to get lost.
I initially had the link to login.php - I ended up changing it for some reason, I don't remember why =/
-
In the loggedin.php, where is the redirect variable specified?
function checkLogin($redirect)
{
// would check if the correct $_SESSION variable is set. If so, user is valid
// and can view the requested page. If not, redirect the user using the header()
// function to whatever $redirect is set to
if (!isset($_SESSION['loggedin']))
{
// redirect user
header("location: " . $redirect);
I'm assuming I should be replacing "location:" with the path to the page in which I'd like to redirect?
Also, does not seem to be logging in with username/pass, but the form seems to be in tact.
The page is very basic, but as I've mentioned, I'm entirely new to all of this, you can find the URL here"
http://cis166.estrellamountain.edu/DARUP97011/module8.php
Click the "login page" link on the nav bar to get to the form.
-
Thanks a crap ton. Going to try to implement this code with some modifications, may take me a bit of time (by this evening for sure, the school website is having issues so I can't access the ftp to upload data). Ideally I'd like to understand what I am doing as I add it in, otherwise this course was a waste of time
Your comments in the code appear to explain the whole process, I greatly appreciate the help! I'll post results and link to the page when completed.
Thanks again!
-
I am taking a beginning course in PHP, just as I thought I was getting the hang of things, the course threw in some OPP. Granted I've never really touched programming before, this is all new to me. The course seems to go from basic to "I'm lost as hell" in about 2 seconds. That being said, the course requires this:
* Create an LoginBox object class with all of the functionality needed to authenticate a user and redirect to another page based on success or failure
* A possible list of properties could be Action, Title, ButtonText, SuccessRedirect, FailRedirect, etc
I've watched all the killerphp videos on OPP, so I have a VERY basic understanding of how objects/classes work. However, the course does not provide any guidance on authentication methods or anything related to user authentication via php.
I figure I'll have a form page, in that form it will have two fields (username, password). The password can be the same for all users, and the password field may not be required at all. Basically, you have to put in a username/pass that exists in the class/object and this will re-direct you to the main page. If you do not authenticate, it will re-direct you to another page.
So far, I'll be needing:
-Basic php form page with 2 fields and a button
-A class that contains all the methods for what the object will do
-A button (object), when clicked, references the class and performs necessary checks for authentication
Does this seem right?
In all honesty, I really don't have a clue where to begin. While I do find all of this interesting, I feel I'm in over my head -- at least at this point.
Any guidance would be GREATLY appreciated.
Thanks,
Darren
Authenticate and Redirect using Object Oriented PhP - HOW????
in PHP
Posted
On the last module!
I am just trying to get the NSlookup function to work, it will work if I do not use the $_GET variable. If I just specify a domain (cnn.com) it will work fine. if I try to request data, the page thinks until it times out.
Form data:
The problem I think I am having is this:
The passthru command sends the specified line to the server (i.e. not running it in php any longer). Since the actual server has no clue what $_GET is, it simply displays the results of nslookup and does not display the information from the form. That being said, what I need to do is have the text from the form be input into the pass through command before it is executed on the server. Maybe something like this:
Type domain in form --> submit --> php takes $_GET["nslookup"] data and then places the data into passthru("nslookup xxxxxx") where xxxxx is the value of $_GET["nslookup"] --> server executes command --> data displayed on page
Any ideas?