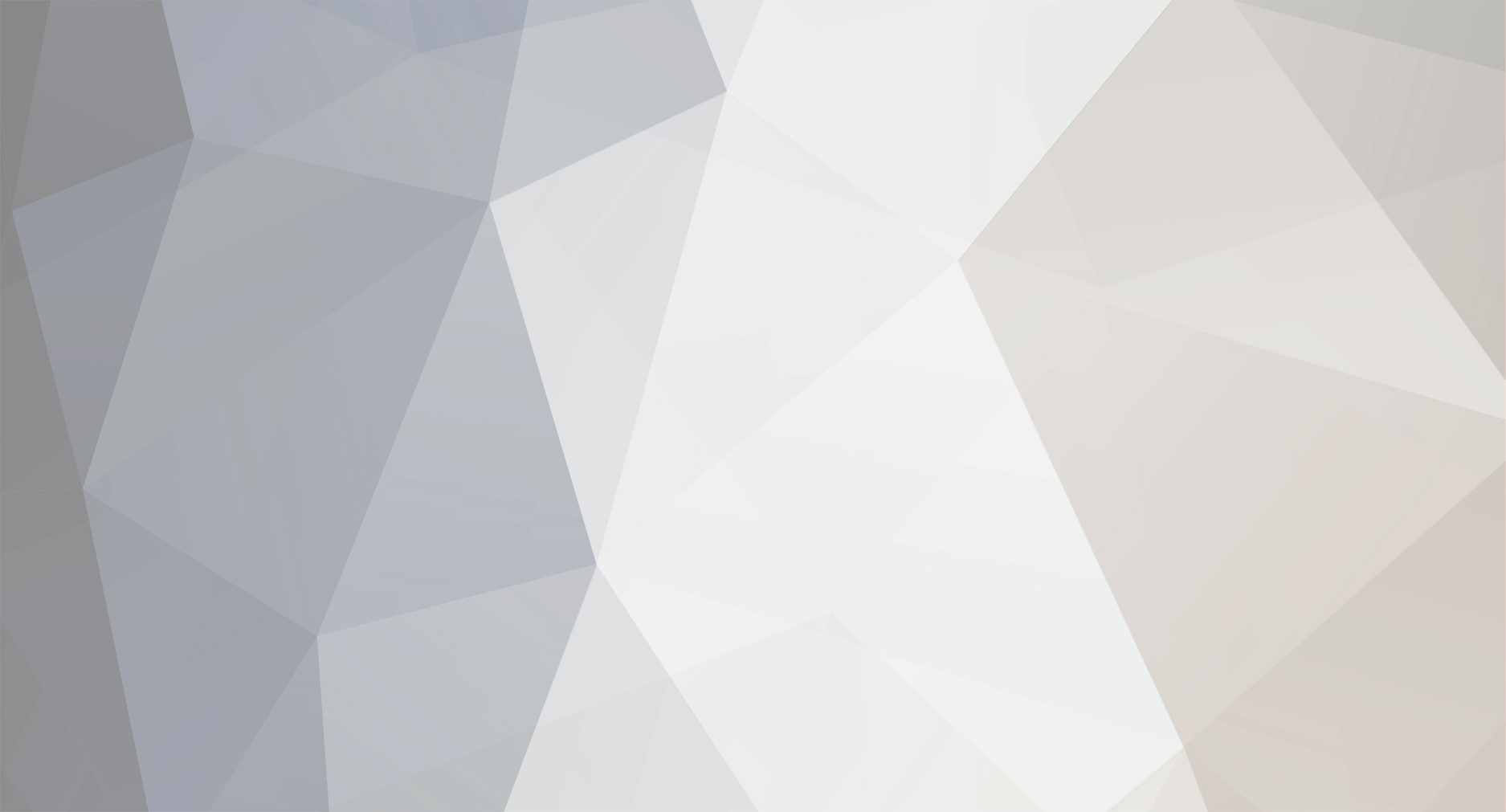
SubhanUllah
Member-
Posts
132 -
Joined
-
Last visited
Content Type
Profiles
Forums
Events
Downloads
Gallery
Store
Everything posted by SubhanUllah
-
I have never used nested interfaces. The only case I can see where this is sensible is the multi-layered Sealed Interface pattern, e.g. interface Event { // definitions interface Foo extends Event { // Foo-specific event definitions } interface Bar extends Event { // Bar-specific event definitions } }
-
int a; int *b = &a; Here the b is a pointer. It has been assigned value &a, which means the-address-of-a.
-
Curly braces are used for initialization of objects. If the object supports it the members (storage) of the object will be directly assigned to the values in the braces. std::string s{"a string"}; int a[] = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 20 }; struct X { int x; int y; int z; }; X x{ 1, 3, 5}; X y = { 4, 5, 6}; This is called Unified Initialization, so that there is a consistent syntax, based around curly braces: int i{}; // initialized built-in type, equals to int i{0}; int j{10}; // initialized built-in type int a[]{1, 2, 3, 4} // Aggregate initialization X x1{}; // default constructor X x2{1}; // Parameterized constructor; X x4{x3}; // copy-constructor int* arr = new int[5]{ 1, 2, 3, 4, 5 }; //dynamic allocation with initialization
-
How to reverse a string in C++ without a function?
SubhanUllah posted a topic in General Programming
Here is how to reverse a string without a function: #include <iostream> #include <cstring> using namespace std; int main() { int i, n = 123456789, j, k, l, strlen; char str[10*(sizeof(char))]; char newstr[sizeof(str)]; bool is_positive = true; k=sizeof(str)/sizeof(str[0]); if (k < 0 ) { is_positive = false; } if (k < 0 ) is_positive = false; sprintf(str,"%d",n); //converts to decimal base. cout << " k is " << k << " str is " << str << endl; if (!is_positive) exit(1); j = 0; l = k-2; i = 0; while(i++ <= k){ /*cout << "newstr[" << j << "] is " << newstr[j] << " str[" << l << "] is " << str[l] << endl; */ newstr[j++] = str[l--]; } cout << "newstr is " << newstr << endl; return 0; } Outputs k is 10 str is 123456789 newstr is 987654321 Without using reverse of swap functions. -
<h1></h1> is the largest heading tag in HTML. But,it can be customized by CSS to make it more large. Example : <!DOCTYPE html> <html> <head> <style> h1{ font-size: 62px; } </style> </head> <body> <h1>The table element</h1> </body> </html>
-
Add options to a select box in JavaScript: 1) Using the Option constructor and add() method: First, use the Option constructor to create a new option with the specified option text and value: let newOption = new Option('Option Text','Option Value'); Then, call the add() method of the HTMLSelectElement element: const select = document.querySelector('select'); select.add(newOption,undefined); 2) Using the DOM methods First, construct a new option using DOM methods: // create option using DOM const newOption = document.createElement('option'); const optionText = document.createTextNode('Option Text'); // set option text newOption.appendChild(optionText); // and option value newOption.setAttribute('value','Option Value'); Second, add the new option to the select element using the appendChild() method: const select = document.querySelector('select'); select.appendChild(newOption);
-
It's an array of strings. Example: #include <string> #include <iostream> #include <array> int main() { std::array<std::string, 2> a = {"Hello ", "world!"}; std::cout << a[0] << a[1]; } Here the a is an array of strings.
-
Two equal signs is a comparison. So: $a=1; Sets $a to 1. Now we can use it to test. If ($a==1) echo ‘A equals 1’; If (!$a!==1) echo ‘A does not equal 1’; You do have other ways of using this: >= check for greater than or equal to !>= not greater than or equal to. And the same for lesser than.
-
Assuming each URL is a .php file then set the PHP file yo: <?php header (‘Location: /path/to/newUrl.php'); Exit(); If you have one PHP script with different get data: <?php # this is index.php. you call it with # index.php?page=1 # to # index.php?page=4 # you should do this with case, but this works. If ($_GET[‘page']==1) { header (‘Location: /path/page1.php'); exit (); } If ($_GET[‘page']==2) { header (‘Location: /path/page2.htm'); exit (); } If ($_GET[‘page']==3) { header (‘Location: /path/page3.html'); exit (); } If ($_GET[‘page']==4) { header (‘Location: https://new.domain. com'); exit (); }
-
XML doesn’t tolerate syntax errors. HTML does, with specifications on to correct them. XML tags have to be closed. They can auto-close, like <stuff/> which is equivalent to <stuff></stuff>. HTML tags that are meant to be empty, don’t need a syntax that closes them. XML tags’ attribute values have to be quote. It’s <a thing="stuff"> or <a thing='stuff'> but not <a thing=stuff> XML tags don’t tolerate < other than to open a tag. If you want the text < in XML you need < . In HTML you can use < if it doesn’t look like a correct tag opening. That’s just the obvious. There are many others.
-
Randint() and shuffle are your friends here. import random test_list = [random.randint(-100000, 100000) for i in range(300)] print(f"300 Random Ints \n{test_list}") # shuffle() mixes the list in place second_list = list(range(40)) random.shuffle(second_list) print(f"First 40 Ints shuffled \n{second_list}") # sample returns a new list and leaves the old one alone string_list = "Tell me and I forget, teach me and I may remember, involve me and I learn. Benjamin Franklin".split() shuffled_words = random.sample(string_list,len(string_list)) print(f"Original words \n{string_list}") print(f"And sample() to shuffle\n{shuffled_words}") The random.randint() and range() creates a list of 300 numbers selected randomly, from -100,000 to 100,000. Just change the parameters to get what you want. The second shuffles any list not just Integers. Sample() also shuffles and existing list, but creates a new list.
-
While defining the function or class, in the parentheses (), write the parameter followed by a =, followed by the default value… class Circle(): pi = 3.14 def __init__(self, radius=2): self.radius = radius def area(self): return self.pi * (self.radius)**2 c = Circle() print(c.area()) #OUTPUT 12.56
-
I would use a function But you can just put the code anywhere def get_pos_int(prompt='Please enter a positive Integer:'): while True: inp = input(prompt) try: num = int(inp) except ValueError: print(f"{inp} was not an Integer") continue else: if num < 0: print(f"{num} is not a positive Integer") continue else: break return num a = 1 + get_pos_int("Please enter a number to add to one:") print(f"result was {a}") b = get_pos_int() print (f"got {b} back") Output python get_positive.py Please enter a number to add to one:-12 -12 is not a positive Integer Please enter a number to add to one:A A was not an integer Please enter a number to add to one:4 result was 5 Please enter a positive Integer:10 got 10 back
-
Code Example : <html> <head> <style> h1{ font-size: 8px; } </style> </head> <body> <h1>Changing 1st heading size by Internal CSS<h1> <h1 style=”font-size:14px;”>Changing Second heading size by Inline CSS<h1> </body> </html>
-
The calc() CSS function lets you perform calculations when specifying CSS property values. It can be used anywhere a <length>, <frequency>, <angle>, <time>, <percentage>, <number>, or <integer> is allowed. Example: width: calc(100% - 80px); width: calc(10px + 100px); width: calc(100% - 30px); width: calc(2em * 5); width: calc(var(--variable-width) + 20px); Usage with integers: .modal { z-index: calc(3 / 2); }
-
Here is the HTML & CSS code for transparent button: <!DOCTYPE html> <html> <head> <style> button { background-color: #65d7f521; padding: 10px 20px; border: none; color: #00000091; } </style> </head> <body> <button>Button</button> </body> </html>
-
Try to parse it and catch any failure. There isn’t a more fool-proof way to check if JSON is valid than that. Something like this would work: function isValidJSON(s) { try { JSON.parse(s); return true; } catch (e) { } return false; } // Run a few test cases: console.log(`isValidJSON("4") = ${isValidJSON("4")}`); // prints true console.log(`isValidJSON("[4") = ${isValidJSON("[4")}`); // prints false console.log(`isValidJSON("[4]") = ${isValidJSON("[4]")}`); // prints true
-
Here is the HTML & CSS code for curved text: <!DOCTYPE html> <html lang="en"> <head> <!--Style to transform text in an arc --> <style type=text/css> /* Apply the translate and rotate transformation for our text to look curved */ .Q { transform: translate(20px, 85px) rotate(-30deg); } .u { transform: translate(13px, 55px) rotate(-25deg); } .o { transform: translate(6px, 35px) rotate(-20deg); } .r { transform: translate(3px, 23px) rotate(-15deg); } .a { transform: translate(2px, 14px) rotate(-10deg); } .A { transform: translate(1px, 8px) rotate(-5deg); } .n { transform: translate(0px, 5px) rotate(0deg); } .s { transform: translate(-1px, 8px) rotate(5deg); } .w { transform: translate(-2px, 14px) rotate(10deg); } .e { transform: translate(-3px, 25px) rotate(15deg); } .e { transform: translate(-6px, 30px) rotate(20deg); } .r1 { transform: translate(-14px, 50px) rotate(25deg); } .s1 { transform: translate(-24px, 68px) rotate(29deg); } /* An inline-block element is placed as an inline element (on the same line as adjacent content), but it behaves like a block element */ span { display: inline-block; } </style> </head> <body> <div style="text-align: center; padding-top: 250px; font-size: 55px; color: green;"> <!-- Declare all the characters of text one-by-one, inside span tags --> <span class="Q">Q</span> <span class="u">u</span> <span class="o">o</span> <span class="r">r</span> <span class="a">a</span> <span class="A">A</span> <span class="n">n</span> <span class="s">s</span> <span class="w">w</span> <span class="e">e</span> <span class="r1">r</span> <span class="s1">s</span> </div> </body> </html>
-
<img src=”NameOfThePic”> If it's in a different folder it's the same but with <img src=“FolderName/NameOfPic”>