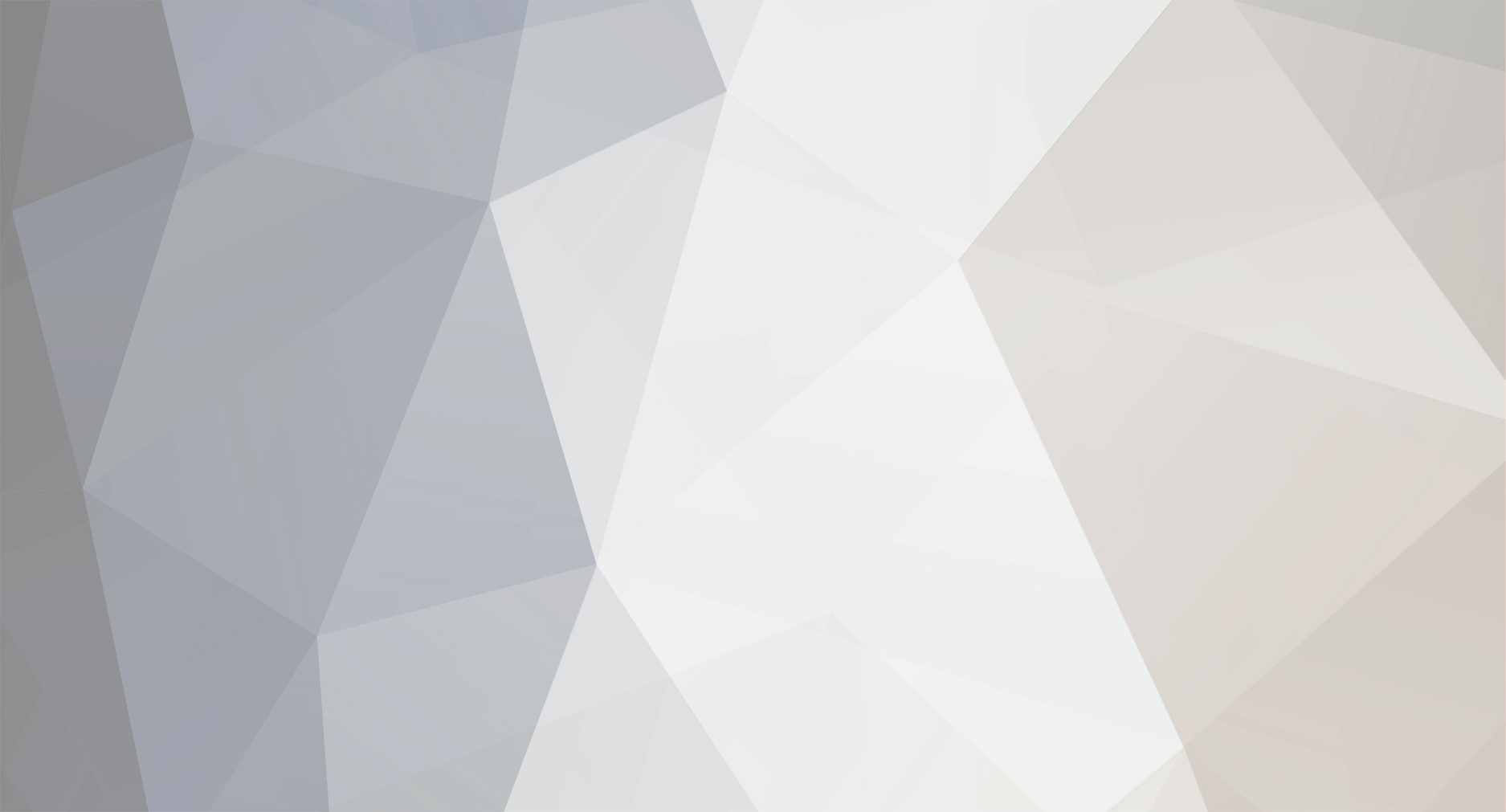
SubhanUllah
Member-
Posts
132 -
Joined
-
Last visited
Content Type
Profiles
Forums
Events
Downloads
Gallery
Store
Everything posted by SubhanUllah
-
In C the result for the variable is the same after the instruction. The difference is where you put the ++; in front or behind the variable. so a=0 a++ ++a a+=1 make a = 3 a = 0 b = a++ // c is assigned first and is 0, then a becomes 1 c = ++a // a becomes 2 then c is assigned the 2 a += 1 // a becomes 3 Make a = 3 but b = 0 and c = 2 , When the ++ is last, the assignment is done first then the value of ‘a’ is bumped by one. Without the equal sign they are all the same; ‘a’ goes up by one.
-
For numbers its pretty easy. Addition is reversible. For other types of data, not to much: #include <cstdio> int main() { int a = 123; int b = 44; float c = -2.234; float d = 4.456; printf("%-18s %11s %14s\n"," ","a <-> b","c <-> d"); printf("%-15s %7d\t%7d\t%7.3f\t%7.3f\n","Starting values",a,b,c,d); a = a + b; // a has both numbers c = c + d; // c now is both numbers printf("%-15s %7d\t%7d\t%7.3f\t%7.3f\n","a = a + b",a,b,c,d); b = a - b; // b now has a d = c - d; // d now has c printf("%-15s %7d\t%7d\t%7.3f\t%7.3f\n","b = a - b",a,b,c,d); a = a - b; // a now has b c = c - d; // c now had d printf("%-15s %7d\t%7d\t%7.3f\t%7.3f\n","a = a - b",a,b,c,d); } Output: a <-> b c <-> d Starting values 123 44 -2.234 4.456 a = a + b 167 44 2.222 4.456 b = a - b 167 123 2.222 -2.234 a = a - b 44 123 4.456 -2.234
-
<!DOCTYPE html> <html> <head> <link href="style1.css" rel="stylesheet"> <link href="style2.css" rel="stylesheet"> </head> <body> </body> </html>
-
<!DOCTYPE html> <html> <head> <meta name="viewport" content="width=device-width, initial-scale=1"> <style> .responsive { width: 100%; height: auto; } </style> </head> <body> <h2>Responsive Images</h2> <p>If you want the image to scale both up and down on responsiveness, set the CSS width property to 100% and height to auto.</p> <p>Resize the browser window to see the effect.</p> <img src="img_nature.jpg" alt="Nature" class="responsive" width="600" height="400"> </body> </html>
-
<!DOCTYPE html> <html> <head> <style> div.a { line-height: normal; } div.b { line-height: 1.6; } div.c { line-height: 80%; } div.d { line-height: 200%; } </style> </head> <body> <h1>The line-height Property</h1> <h2>line-height: normal (default):</h2> <div class="a">This is a paragraph with a standard line-height.<br> The standard line height in most browsers is about 110% to 120%.</div> <h2>line-height: 1.6 (recommended):</h2> <div class="b">This is a paragraph with the recommended line-height.<br> The line height is here set to 1.6. This is a unitless value;<br> meaning that the line height will be relative to the font size.</div> <h2>line-height: 80%:</h2> <div class="c">This is a paragraph with a smaller line-height.<br> The line height is here set to 80%.</div> <h2>line-height: 200%:</h2> <div class="d">This is a paragraph with a bigger line-height.<br> The line height is here set to 200%.</div> </body> </html>
-
<!DOCTYPE html> <html> <head> <style> body { background-color: linen; } h1 { color: maroon; margin-left: 40px; } </style> </head> <body> <h1>This is a heading</h1> <p>This is a paragraph.</p> </body> </html>
-
import os full_path = '/home/User/Documents/file.txt' head_tail = os.path.split(full_path) print (f"The full path is '{full_path}’") print (f"The path is '{head_tail[0]}'") print(f"and the file name is '{head_tail[1]}’ ") output: The full path is '/home/User/Documents/file.txt’ The path is '/home/User/Documents' and the file name is 'file.txt’
-
In C : char string[100]; ... string[27] = 'A'; In C++ vector<char> string; ... string.put(27, 'A'); In Java: char string[100]; ... string[27] = 'A';
-
#include <iostream> #define r { #define b int #define g = #define m >> #define q for #define f , #define h ; #define n :: #define s cout #define p std #define i } #define j main #define k ( #define l << #define t cin #define o ) b j k o r b a f c f e g 0 h q k a g 1 h h c g 3 o r p n t m a h e g e + a h p n s l "\123\x75\155\x3a\40" l e l "\12" h i i
-
Can multiple .PHP files be included in a single .HTML page?
SubhanUllah posted a topic in HTML/XHTML
Yes, possible by including , here is the sample code: <!DOCTYPE html> <html> <body> <?php include 'header.php';?> <h1>Welcome to my home page!</h1> <p>Some text.</p> <?php include 'body.php';?> <p>Some more text.</p> <?php include 'footer.php';?> </body> </html> -
Check the block, in which the variable is declared. When the block ends, the scope ends, and the variable is not valid any more. Example: if (1) { int a; // scope starts if (1) { } // scope ends }