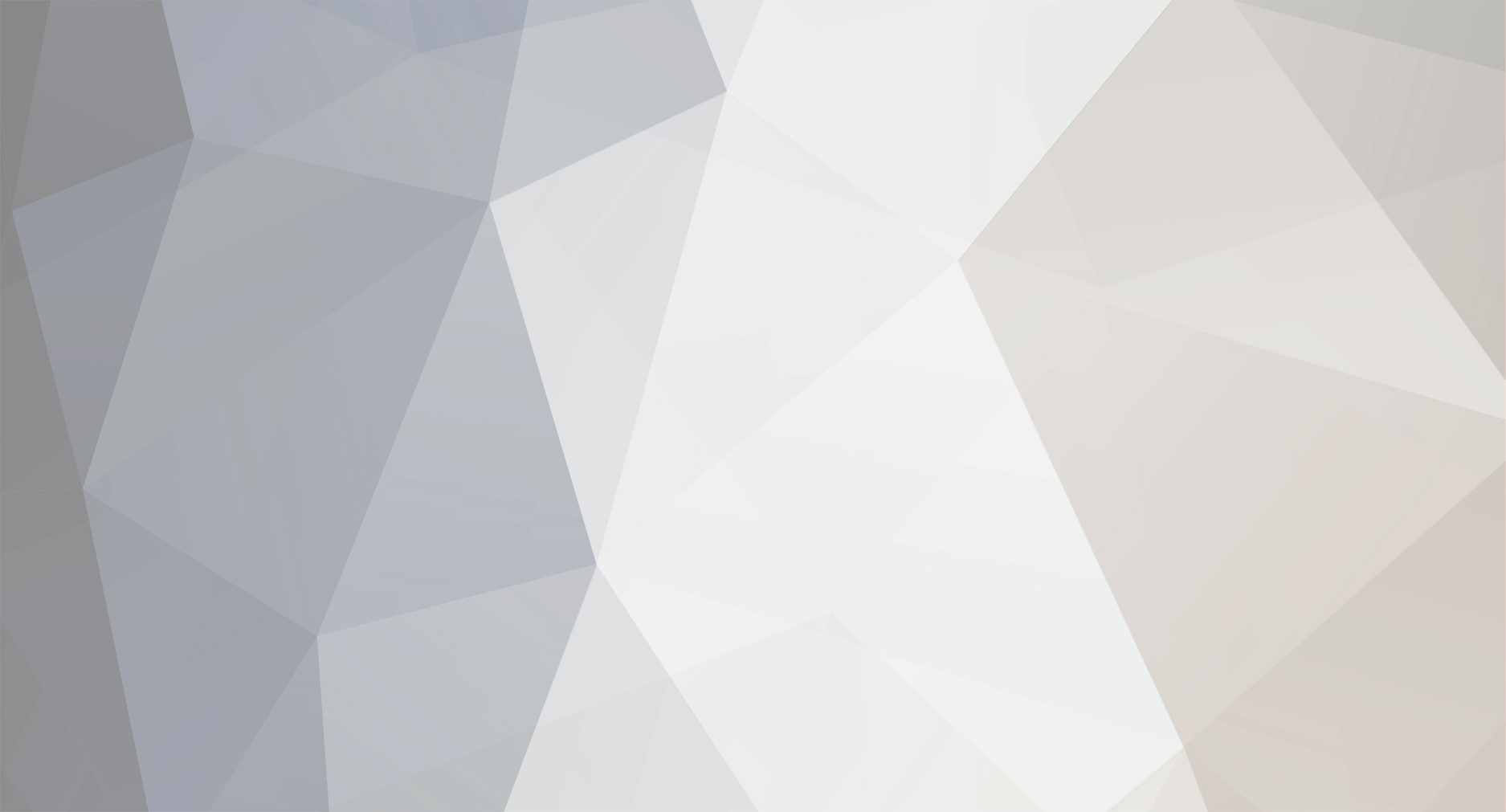
SubhanUllah
Member-
Posts
132 -
Joined
-
Last visited
Content Type
Profiles
Forums
Events
Downloads
Gallery
Store
Everything posted by SubhanUllah
-
<!DOCTYPE html> <html> <head> <!-- CSS --> <link rel="stylesheet" href="https://unpkg.com/flickity@2/dist/flickity.min.css"> <!-- JavaScript --> <script src="https://unpkg.com/flickity@2/dist/flickity.pkgd.min.js"></script> </head> <body> <?php $db = mysqli_connect("localhost", "root", "", "post_images"); $result = mysqli_query($db, "SELECT * FROM posts"); ?> <?php while ($row = mysqli_fetch_array($result)) : ?> <div class="post_container">$row['post_title']; <?php $resultx = mysqli_query($db, "SELECT img_file, img_title FROM images WHERE post_id = " .$row['id_post']); ?> <?php if (mysqli_num_rows($resultx) == 1) : ?> <div class="image_container"> <div class="post_image_displayer"> <?php while ($rowx = mysqli_fetch_array($resultx)) : ?> <img src='../folder_image_uploads/<?php echo $rowx['img_file']; ?>'><?php echo $rowx['img_title']; ?> <?php endwhile; ?> </div> </div> <?php elseif(mysqli_num_rows($resultx) > 1) : ?> <div class="main-carousel" data-flickity='{ "cellAlign": "left", "contain": true }'> <?php while ($rowx = mysqli_fetch_array($resultx)) : ?> <div class="carousel-cell"><img src='../folder_image_uploads/<?php echo $rowx['img_file']; ?>' ></div> <?php endwhile; ?> </div> <?php endif; ?> </div> <?php endwhile; ?> </body> </html>
-
Use count() or sizeof() as follows: $array = [1, 2, 3]; $array_size = count($array); // 3 // or $array_size = sizeof($array); // 3
-
... ... //define you structure type typedef struct { ...... } MyStruct; //now, the array of pointes #define MAX_MYSTRUCTS 200 MyStruct *pointers[MAX_MYSTRUCTS]; // As you dont have space to hold your structures, you might use malloc to allocate // each new created structure. So you will also need a counter to know how many // structures you have created. int nMyStruct = 0; // You can make a function to add a new struct int addMyStruct() { void tempPointer; // check if there is space in the array for new pointer if(nMyStruct>=MAX_MYSTRUCT) return -1; //Ask malloc for space for 1 structure tempPointer = malloc(sizeof(MyStruct)); //if pointer is null, malloc couldnt do it if(tempPointer==NUL) returen -1; //Else store the pointer and recast it pointers[nMyStruct] = (MyStruct *)tempPointer ; //return the place it was stored and add 1 to nMyStruct return nMyStruct++; }
-
my_list = ["A","B","C","Dummy"] junk_list = [] while True: new_value = input("Enter a value 'quit' to end:") if new_value == "quit": break if new_value.isalpha(): my_list.append(new_value) else: junk_list.append(new_value) print(f"Alpha list is: {my_list}") print(f"Junk List is: {junk_list}") Output Enter a value 'quit' to end:dddd Enter a value 'quit' to end:tttt Enter a value 'quit' to end:555 Enter a value 'quit' to end:@#44 Enter a value 'quit' to end:quit Alpha list is: ['A', 'B', 'C', 'Dummy', 'dddd', 'tttt'] Junk List is: ['555', '@#44']
-
<!DOCTYPE html> <html> <head> <meta name="viewport" content="width=device-width, initial-scale=1"> <style> table { border-collapse: collapse; border-spacing: 0; width: 100%; border: 1px solid #ddd; } th, td { text-align: left; padding: 8px; } tr:nth-child(even){background-color: #f2f2f2} </style> </head> <body> <h2>Responsive Table</h2> <p>If you have a table that is too wide, you can add a container element with overflow-x:auto around the table, and it will display a horizontal scroll bar when needed.</p> <p>Resize the browser window to see the effect. Try to remove the div element and see what happens to the table.</p> <div style="overflow-x:auto;"> <table> <tr> <th>First Name</th> <th>Last Name</th> <th>Points</th> <th>Points</th> <th>Points</th> <th>Points</th> <th>Points</th> <th>Points</th> <th>Points</th> <th>Points</th> <th>Points</th> <th>Points</th> </tr> <tr> <td>Jill</td> <td>Smith</td> <td>50</td> <td>50</td> <td>50</td> <td>50</td> <td>50</td> <td>50</td> <td>50</td> <td>50</td> <td>50</td> <td>50</td> </tr> <tr> <td>Eve</td> <td>Jackson</td> <td>94</td> <td>94</td> <td>94</td> <td>94</td> <td>94</td> <td>94</td> <td>94</td> <td>94</td> <td>94</td> <td>94</td> </tr> <tr> <td>Adam</td> <td>Johnson</td> <td>67</td> <td>67</td> <td>67</td> <td>67</td> <td>67</td> <td>67</td> <td>67</td> <td>67</td> <td>67</td> <td>67</td> </tr> </table> </div> </body> </html>
-
$array = [ [1, 2], [3, 4] ]; $row = $array[1]; /* * Output: * Array( [0] => 3 [1] => 4 ) */ print_r($row);
-
$arrays_array = array(array("foo", "bar"), array("baz", "quux")); $parent_array_len = count($arrays_array); for($i = 0; $i < $parent_array_len; $i++){ $nested_array = $arrays_array[$i]; $nested_array_len = count($nested_array); for($j = 0; $j < $nested_array_len; $j++){ /* * Outputs: * foo * bar * baz * quux */ echo $nested_array[$j].PHP_EOL; } }
-
for (size_t i = 0; i < sizeof(array) / sizeof(array[0]); i++) printf(" %d", array[i]);
-
for b in [1, 2, 3, 4, 5]: if b%2==0: print(f"{b} is even.") else: print(f"{b} is not even.") ###OUTPUT 1 is not even. 2 is even. 3 is not even. 4 is even. 5 is not even.
-
<!DOCTYPE html> <html> <head> <style> body { background-color: pink; } @media screen and (min-width: 480px) { body { background-color: lightgreen; } } </style> </head> <body> <h1>Resize the browser window to see the effect!</h1> <p>The media query will only apply if the media type is screen and the viewport is 480px wide or wider.</p> </body> </html>
-
<!DOCTYPE html> <html> <head> <style> #holder { border: 1px solid #000; height: 200px; width: 200px; position:relative; margin:10px; } #mask { position: absolute; top:-1px; left:1px; width:50%; height: 1px; background-color:#fff; } </style> </head> <body> <div id="holder"> <div id="mask"></div> </div> </body> </html>
-
#include <stdio.h> int main( void ) { char greet[] = "Hello"; char* who = "World"; print( "%s, %s!" , greet , who ); return 0; }
-
#include <vector> #include <mdspan> #include <print> int main() { std::vector v = {1,2,3,4,5,6,7,8,9,10,11,12}; // View data as contiguous memory representing 2 rows of 6 ints each auto ms2 = std::mdspan(v.data(), 2, 6); // View the same data as a 3D array 2 x 3 x 2 auto ms3 = std::mdspan(v.data(), 2, 3, 2); // write data using 2D view for(size_t i=0; i != ms2.extent(0); i++) for(size_t j=0; j != ms2.extent(1); j++) ms2[i, j] = i*1000 + j; // read back using 3D view for(size_t i=0; i != ms3.extent(0); i++) { std::println("slice @ i = {}", i); for(size_t j=0; j != ms3.extent(1); j++) { for(size_t k=0; k != ms3.extent(2); k++) std::print("{} ", ms3[i, j, k]); std::println(""); } } }
-
<!DOCTYPE html> <html> <head> <style> img { float: right; } </style> </head> <body> <h1>The float Property</h1> <p>In this example, the image will float to the right in the text, and the text in the paragraph will wrap around the image.</p> <p><img src="pineapple.jpg" alt="Pineapple" style="width:170px;height:170px;margin-left:15px;"> Lorem ipsum dolor sit amet, consectetur adipiscing elit. Phasellus imperdiet, nulla et dictum interdum, nisi lorem egestas odio, vitae scelerisque enim ligula venenatis dolor. Maecenas nisl est, ultrices nec congue eget, auctor vitae massa. Fusce luctus vestibulum augue ut aliquet. Mauris ante ligula, facilisis sed ornare eu, lobortis in odio. Praesent convallis urna a lacus interdum ut hendrerit risus congue. Nunc sagittis dictum nisi, sed ullamcorper ipsum dignissim ac. In at libero sed nunc venenatis imperdiet sed ornare turpis. Donec vitae dui eget tellus gravida venenatis. Integer fringilla congue eros non fermentum. Sed dapibus pulvinar nibh tempor porta. Cras ac leo purus. Mauris quis diam velit.</p> </body> </html>
-
#include <stdio.h> int main(){ int zzz[] = {12,34,56,78,90}; printf("%d\n", sizeof(zzz)/sizeof(int)); }
-
How to create a responsive website using only CSS?
SubhanUllah posted a topic in General Programming
<!DOCTYPE html> <html> <head> <style> body { background-color: pink; } @media screen and (min-width: 480px) { body { background-color: lightgreen; } } </style> </head> <body> <h1>Resize the browser window to see the effect!</h1> <p>The media query will only apply if the media type is screen and the viewport is 480px wide or wider.</p> </body> </html> -
Code sample using the reduce function: const myArrayOfObjects = [ { myKey: 1, data: 'some data' }, { myKey: 2, data: 'some data' }, { myKey: 3, data: 'some data' }, { myKey: 4, data: 'some data' }, { myKey: 5, data: 'some data' }, { myKey: 6, data: 'some data' }, { myKey: 7, data: 'some data' }, ]; const myMap = myArrayOfObjects.reduce( (accumulator, currentObject) => ({ ...accumulator, [currentObject.myKey]: currentObject, }), {} ); console.log(myMap); // output: // { // '1': { myKey: 1, data: 'some data' }, // '2': { myKey: 2, data: 'some data' }, // '3': { myKey: 3, data: 'some data' }, // '4': { myKey: 4, data: 'some data' }, // '5': { myKey: 5, data: 'some data' }, // '6': { myKey: 6, data: 'some data' }, // '7': { myKey: 7, data: 'some data' } // } Repeated keys will be overwritten, so take into account that if you have repeated keys only the last one will remain.
-
To split an array, you can import numpy and use the np.array_split(array, # of splits) method. for example: arr = np.array([1, 2, 3, 4, 5, 6]) newarr = np.array_split(arr, 3) print(newarr) >> [array([1, 2]), array([3, 4]), array([5, 6])]