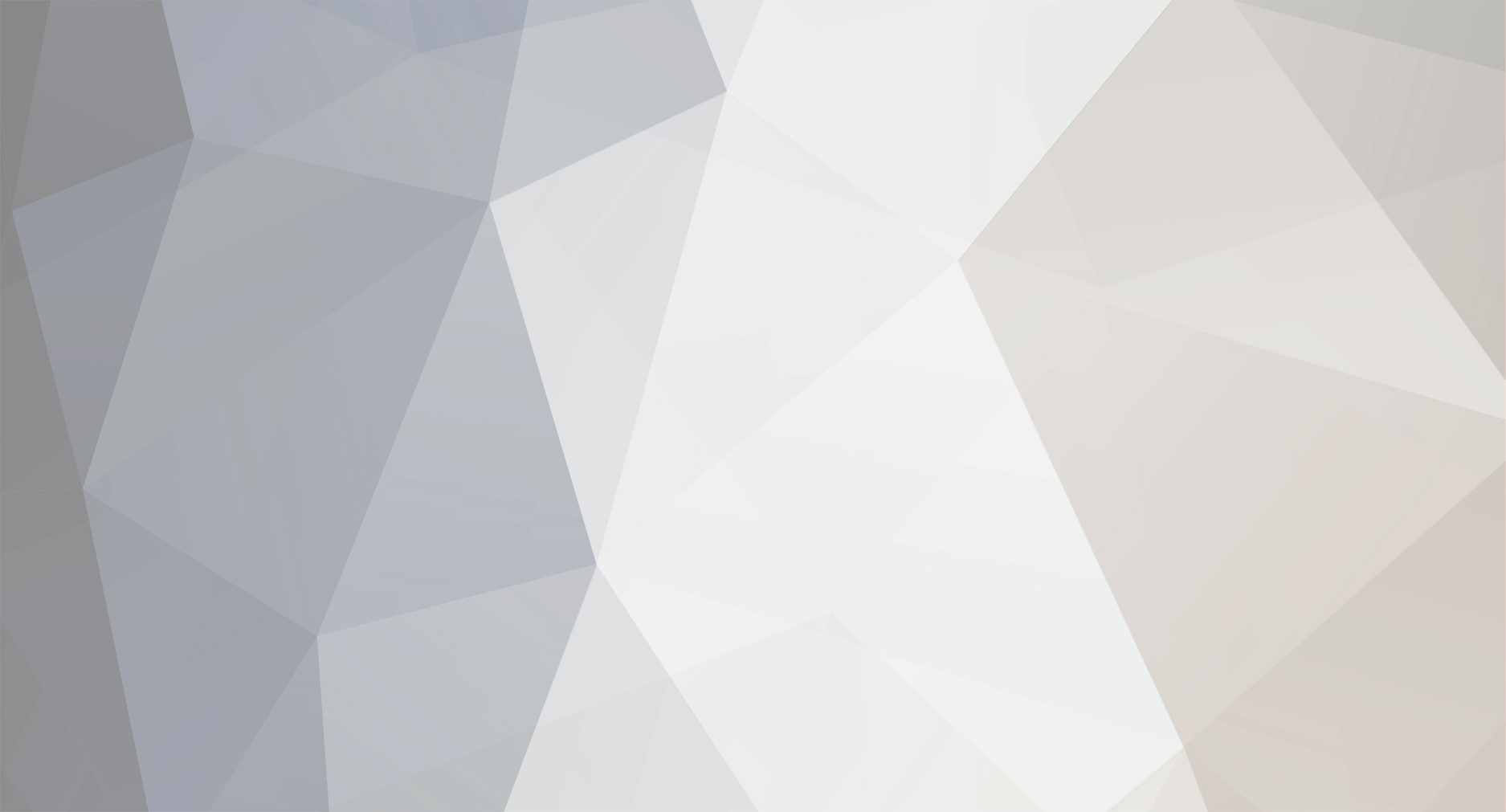
Drew2
-
Posts
26 -
Joined
-
Last visited
-
Days Won
1
Content Type
Profiles
Forums
Events
Downloads
Gallery
Store
Posts posted by Drew2
-
-
Update: actually, this code doesn't quite do it. It does something else. So, I'd say the course could use some updating.
-
Hello Jim,
I understand your frustration.
I was able to get through and understand the CRUD basics course.
Yeah, 2 months is a pretty rough response time.
I assume you've repeated the CRUD course a few times.
If you haven't tried this already, I'd put your code in difchecker (https://www.diffchecker.com/) alongside the code you can download from the course. What I do is find a section that is probably giving trouble - it may be small. I'll copy the code I've written, and then copy the correct course code and paste it alongside one another. I tend to do this only after I've conceptually understood the concepts - but something just isn't working right in code. What usually happens is that I've missed a quotation mark or a paranthesis. Difchecker finds where the difference is.
If there are no differences in your code (and this happened to me before), I find that my database connection may be to blame (the database password may be different for some users depending on what computer they use: mac or pc. my password is 'root' - others' password is just this: ''.)
-
1
-
-
I like to use dif checker: https://www.diffchecker.com/
I copy a section of code that is correct (Ben's code), and then match it against what I have written. I tend to find errors that way.
-
So here seems to be the stages leading up to $_SESSION['cart'] being set:
First, someone clicks a link generated by m_products 'cart.php? id=' . $product['id']
Second, cart.php sees the product id in the url (generated by someone clicking the above link), and calls this function in m_cart.php to add a product: $Cart->add($_GET['id']);
Third, m_cart.php starts this function:
public function add($id, $num = 1)
public function add($id, $num = 1)
{
//setup or retrieve cart
$cart = array();
if(isset($_SESSION['cart']))
{
$cart = $_SESSION['cart'];
}
//check to see if item is already in cart
if (isset($cart[$id]))
{
//if item is in cart
$cart[$id] = $cart[$id] + $num;
}
else
{
// if item is not in cart
$cart[$id] = $num;
}
$_SESSION['cart'] = $cart;
}The section of code above that I have highlighted is where $_SESSION['cart'] is initially set. I was having trouble before, because I hadn't cleared the cart before running the public function add. As a result, every time I tested this line of code, I did so assuming the $_SESSION['cart'] was empty. But when I tested the code the first conditional kept resolving to true, as if the cart had already been set (even though I thought it hadn't been).
At any rate, here is how $_SESSION['cart'] is set. The first two conditionals in public function add resolve to false: $_SESSION['cart'] has not been set yet (provided no previous items were added to the cart). And, if $_SESSION['cart'] hasn't been set yet, there won't be a key of $id in the variable $cart - as $cart is just a blank array. So, that means the last piece of code will fire: a previously blank array ($cart) will be given a key ([$id]) and a value of $num (which is just 1). Then, the information in variable $cart is assigned (given) to $_SESSION['cart'].
-
1
-
-
Got it, thanks
-
Quick question:
Why are there no periods bordering this php insertion?
header("Location: $url");
I would expect it to look like this:
header("Location: . $url . ");
My guess, reading W3Schools and elsewhere is that both would amount to the same thing.
I have to wonder though, it they amount to the same thing, why ever use the periods? -Seems like more work.
-
Hello you wonderful coders you.
I've been staring at this line of code (line 74 highlighted below) for a while in m_template.php, and want to share what it's doing.
function getAlerts()
{
$data = '';
foreach($this->alertTypes as $alert)
{
if (isset($_SESSION[$alert]))
{
foreach($_SESSION[$alert] as $value)
{
$data .= '<li class="'. $alert .'">' . $value . '</li>';
}
unset($_SESSION[$alert]);
}
}
return $data;
}The unset is here so that the next time a user clicks the submit button, the $_SESSION variable will have a clean slate (at least as far as alerts are concerned). Otherwise, a user might click the submit button when they've entered valid information, and receive an error message in the members page.
I just commented out the unset line and ran the code. First I entered wrong information and clicked submit. So $_SESSION now has this in it "you entered an invalid username or password." Then, I entered valid information (so now $_SESSION should also have this in it "You successfully logged in to the members page"). When I did that, I was redirected to the members page. At the top were the alerts. There was an alert saying "You successfully logged in to the members page" and then the error: "you entered an invalid username or password."
Just thought others may have been confused like me about why that line of code is in there at all.
-
1
-
-
I'm on the PHP Logic with OOP section, and have what I think may be a pretty simple question.
In v_login.php, where I see the login form, line 27 for example has this code:
<input type="password" name="password" value="<?php echo $this->getData('input_pass'); ?>"> - This line of code takes the user input, and spits it into the form for the user to see.
Then, in login.php, there is this code on line 30:
$_SESSION['username'] = $Template->getData('input_user'); - this line of code adds to the $_SESSION variable.
As v_login.php is included in login.php, why are there two different ways of calling the getData function? Shouldn't there just be one way to call the function, given v_login.php is included in login.php?
Thanks,
Andrew
-
Hello fellow coder fra168nk. I may have just figured out myself how include works.
Imagine one document with the function of bouncing a ball. (D1)
Then you have a second document with the request to actually bounce a ball (D2)
For D2 to actually bounce a ball, it will need the help of D1. D1 is the tool with which D2 can bounce a ball.
By writing, for example, include(D1) in D2, D2 now has access to the bouncing ball function in D1. D1 and D2 have become one document in a sense (in terms of one benefitting from the other).
Although the documents are now one, in a sense, D1's location (as far as referencing CSS is concerned) is now wherever D2 is located. So, whenever a reference is made in D1 to CSS, it should be made as though D1's location is the same as D2's. It took me a while to figure that one out.
-
Thanks fra168nk! I came to that conclusion as well.
-
1
-
-
Hello,
I'm working through the login System using OOP in Stef's course. In the file m_template.php, there is this line of code:
(Line 58)
function setAlert($value, $type = null)
{
if ($type == '') { $type = $this->alertTypes[0]; }
$_SESSION[$type][] = $value;
}I'm trying to see how the highlighted section of code is working.
Ben explains this, but I don't quite understand yet.
First of all, I want to check that my concept of the session variable $_SESSION is correct:
If I unpack a hypothetical $_SESSION variable, it could look something like this:
$_SESSION [
'success' => ['you are logged in']
'warning' =>[ ]
'error' => []
user => 'John'
]
So, $_SESSION is a variable holding an array. Within that array is another array which could have a key of either success, warning, or error.
$_SESSION might also have other elements in the array like user.
When I write the code $_SESSION[$type][] = $value; I could think of it in this example:
$_SESSION['success'][] = 'you are logged in';
This line of code means that I will be adding to the nested array with the key of 'success'.
So if $_SESSION looked like this instead:
$_SESSION [
'success' => ['logging in is awesome', 'You are so bomb for logging in', 'High Five!', ]
'warning' =>[ ]
'error' => []
user => 'John'
]
I would be tacking on 'you are logged in' like so:
'success' => ['logging in is awesome', 'You are so bomb for logging in', 'High Five!', 'you are logged in']
However, if I left out the brackets like so:
$_SESSION['success'] = 'you are logged in';
then I would over-write the array with the key of 'success' and create this:
'success' => 'you are logged in'
Am I thinking about all of this correctly?
Update: Nov 11, 2020
I wonder if it is a bit unnecessary to have this line of code:
$_SESSION[$type][] = $value (m_template.php, line 58)
rather than this:
$_SESSION[$type]= $value
There doesn't seem to be any circumstance where there is more than one value per key at any given time.
-
I am pouring over the "simple" php login system code for Ben Falk's tutorial. There is one line of code that I cannot make sense of. After reading several sources online about prepared statements, I *only* ever see them with parameters (e.g. ?). Yet, in Ben's tutorial, there is a prepared statement without parameters in file register.php:
// create select options
$select = '<option value="">Select an option</option>';
$stmt = $mysqli->prepare("SELECT id, name FROM permissions");
$stmt->execute();
$stmt->bind_result($id, $name); // for more information, see http://www.php.net/manual/en/mysqli-stmt.bind-result.php
while ($stmt->fetch())
{
$select .= "<option value='" . $id . "'";
if ($input['type'] == $id) { $select .= "selected='selected'"; }
$select .= ">" . $name . "</option>";Why aren't there parameters (e.g. ? ?) in the prepare statement? Isn't that dangerous?
Thanks!
Andrew
Edit: 10.23.2020 - The more I look at the code and think about it, I think it is actually harmless. The drop-down box does not allow users to enter in information, only select it. So, the information being passed to the database couldn't be (for the drop-down box) anything but what is already in the box. Yet, I still find it curious why we are using the prepare statement at all. Why not just mysqli_query(.....)?
-
-
Hello, Same problem for me. I'd like to continue with the course, but can't get past this question. Any suggestions?
-Andrew
-
Got it Thanks :).
-
1
-
-
Gotcha. Thanks!
-Andrew
-
1
-
-
In the course, we are asked to copy and paste code that validates a US phone number. I was unable to find the exact page used in the course.
However, I found another page with code that produces the same result. Here is the page: https://jqueryvalidation.org/phoneUS-method/
Here is the code that makes it all work (the bold portions are what I copy and pasted in):
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01//EN" "http://www.w3.org/TR/html4/strict.dtd">
<html>
<head>
<title>Validation Example</title>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<script type="text/javascript" src="jquery.js"></script>
<script type="text/javascript" src="validate.js"></script>
<script src="https://cdn.jsdelivr.net/jquery.validation/1.16.0/additional-methods.min.js"></script>
<script type="text/javascript">
$(document).ready(function(){
jQuery.validator.setDefaults({
debug: true,
success: "valid"
});
$( "#phone" ).validate({
rules: {
field: {
required: true,
phoneUS: true
}
}
});
$("#form").validate();
});
</script> -
Adding PHP Validation: Chapter 1 lesson 3
minutes: 2:30 -nutshell: If I understand Ben, he adds the php to the input tags in form.php so that when I enter my information in the form and hit submit, my information won't disappear. But, when I take all of the php out that Ben seems to use to keep my information from disappearing, I get the same result: my information stays there. Am I missing something?
I have deleted temporarily all of the php echoes in the input tags in form.php (e.g. I deleted <?php echo $error['name'] ?> ). After I saved the page, I then refreshed the web page with the form. I entered incorrect login information in the form, and clicked submit. My incorrect login information was *still there. As such, I don't yet see the point of adding the php echoes.
-
https://developers.google.com/fonts/faq - found that. It says "The page load time indicator, located in the upper-right of the selection drawer, shows an estimation of how your selection will affect the overall load time (ex: slow, moderate, fast) of your page based on the number of families, styles, and scripts you’ve chosen in the “Customize” tab."
It all sounds great, until I look for it. I've clicked on everything I can think of in the top right, and no mention of the page load time indicator is to be found.
Best,
Andrew
-
In the video there is a page which has a page load indicator, with colors of green, yellow, and red (It sort of looks like a clock). If I remember, the colors indicate how fast the font will load. I cannot find the webpage on Google Fonts with that indicator, nor anything else having to do with the page load speed of any of the Google Fonts.
-
Hello Stefan,
I've been looking for the past 15 minutes for the page load indicator in Google Fonts. I can't find anything on Stack Overflow about the page load indicator in Google Fonts. I also haven't found anything in Google Font's FAQ on this. Any idea what is going on? It seems like font speed is important.
Thank you for your assistance
-Andrew
-
Great! Got em'
Thanks
-
1
-
-
Hello,
I recently purchased the Complete Freelancer Videos, but all I found were mp3s.
Pretty sure I followed the email instructions correctly. Any idea what is going on?
-Drew
CRUD basics with Mysqli & PHP part2: undefined property error.
in StudioWeb Projects
Posted
Yeah, I think it's all about constant practice - which can be tough given there are several coding languages.
I've been told that young monks re-read holy texts tons of times in order to memorize them. So, perhaps taking this course once is good, but maybe not enough to reinforce everything to a master degree.