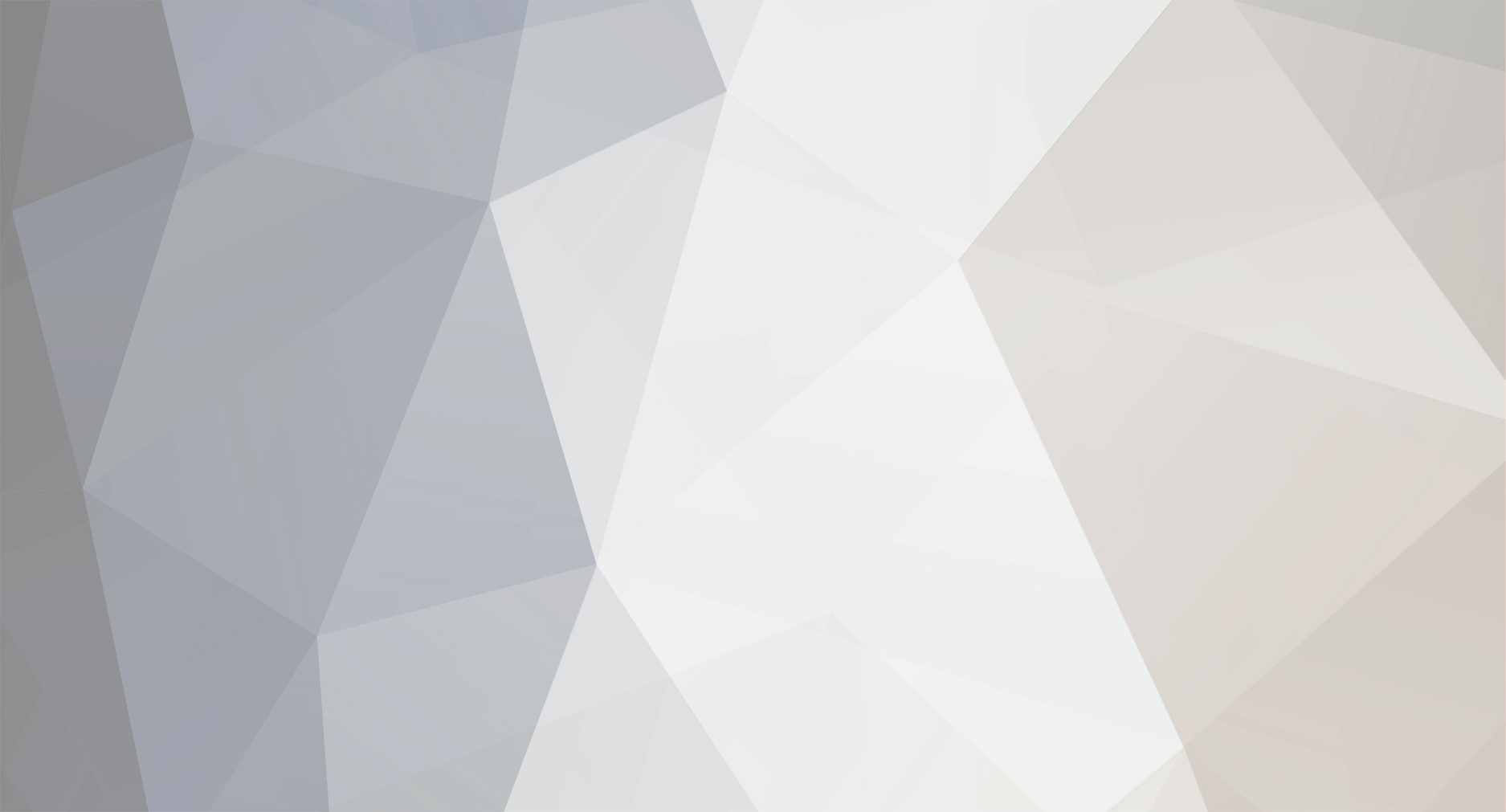
scampbell70
-
Posts
9 -
Joined
-
Last visited
Content Type
Profiles
Forums
Events
Downloads
Gallery
Store
Posts posted by scampbell70
-
-
The check box doesn't stay checked. Is there a way to fix that?
-
I got it working without any errors on my xampp setup by changing
$Active = (int)$_POST['Active'];
to
$Active = !empty($_POST['Active']) ? 'yes' : 'no';
-
I actually tried that example before I posted. I'm sure I'm doing something wrong and probably very simple. I learn best by doing things relevant to something I enjoy but I've only been at this web stuff a few weeks
-
I have received so much help from this site and the amazing people on it. I am humbled by the amount of knowledge everyone here has. I am hoping that you can help me with something that I am struggling to figure out. I have a check box that successfully writes to my db but I can only get it to work with 1 and 0. I would like checked = yes unchecked to = no but I cant figure it out. Here is the code I am currently using.
<strong>Active: *</strong> <input type="checkbox" name="Active" value="1" <?php if($Active == 1) echo 'checked="checked"'; ?>"/><br/>
and
$Active = (int)$_POST['Active'];
-
Is it possible to have 2 or 3 tables in a database example
Table 1 = names
Table 2 = address
Table 3 = phone numbers
and when I fill in my name and number on a form it writes the name to the name table and number to the number table and then I can build a query to pull the phone that goes with the right name?
Is it better to spilt it up this way for security or it doesn't really matter? I just feel like I have way to many rows in a single table on my database.
-
That worked thank you so much!
-
I am using the code from Basic PHP System: View, Edit, Add, Delete records with MySQLi and I am wanting to add some code that will display a total number of results returned from a query.
if ($result = $mysqli->query("SELECT * FROM clients where (`clients`.`aftercare` = 'yes')ORDER BY LastName ASC"))
My question is how can I display how many records are returned. So for example I run it and it returned 40 names. I would like to add something to the page that says 40 people found. I am sure this is probably very simple, but I am brand new to all of this. Thank you for you help in advance.
-
I am getting the following error and I am not sure how to fix it. Warning: mysqli_stmt::bind_result(): Number of bind variables doesn't match number of fields in prepared statement in /Applications/XAMPP/xamppfiles/htdocs/test/records.php on line 135
<?php
/*
Allows the user to both create new records and edit existing records
*/
// connect to the database
include("connect-db.php");
// creates the new/edit record form
// since this form is used multiple times in this file, I have made it a function that is easily reusable
function renderForm($first = '', $middle = '', $last = '', $ClientID = '', $Diagnosis = '', $Gender = '', $LevelCare = '', $Counselor = '', $error = '', $id = '')
{ ?>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01//EN" "http://www.w3.org/TR/html4/strict.dtd">
<html>
<head>
<title>
<?php if ($id != '') { echo "Edit Record"; } else { echo "New Record"; } ?>
</title>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8"/>
</head>
<body>
<h1><?php if ($id != '') { echo "Edit Record"; } else { echo "New Record"; } ?></h1>
<?php if ($error != '') {
echo "<div style='padding:4px; border:1px solid red; color:red'>" . $error
. "</div>";
} ?>
<form action="" method="post">
<div>
<?php if ($id != '') { ?>
<input type="hidden" name="id" value="<?php echo $id; ?>" />
<p>id: <?php echo $id; ?></p>
<?php } ?>
<strong>First Name: *</strong> <input type="text" name="FirstName"
value="<?php echo $first; ?>"/><br/>
<strong>Middle Name: *</strong> <input type="text" name="MiddleName"
value="<?php echo $middle; ?>"/>
<strong>Last Name: *</strong> <input type="text" name="LastName"
value="<?php echo $last; ?>"/>
<strong>Client ID: *</strong> <input type="text" name="ClientID"
value="<?php echo $ClientID; ?>"/>
<strong>Diagnosis: *</strong> <input type="text" name="Diagnosis"
value="<?php echo $Diagnosis; ?>"/>
<strong>Gender: *</strong> <input type="text" name="Gender"
value="<?php echo $Gender; ?>"/>
<strong>Level of Care: *</strong> <input type="text" name="LevelCare"
value="<?php echo $LevelCare; ?>"/>
<strong>Counselor: *</strong> <input type="text" name="Counselor"
value="<?php echo $Counselor; ?>"/>
<p>* required</p>
<input type="submit" name="submit" value="Submit" />
</div>
</form>
</body>
</html>
<?php }
/*
EDIT RECORD
*/
// if the 'id' variable is set in the URL, we know that we need to edit a record
if (isset($_GET['id']))
{
// if the form's submit button is clicked, we need to process the form
if (isset($_POST['submit']))
{
// make sure the 'id' in the URL is valid
if (is_numeric($_POST['id']))
{
// get variables from the URL/form
$id = $_POST['id'];
$FirstName = htmlentities($_POST['FirstName'], ENT_QUOTES);
$MiddleName = htmlentities($_POST['MiddleName'], ENT_QUOTES);
$LastName = htmlentities($_POST['LastName'], ENT_QUOTES);
$ClientID = htmlentities($_POST['ClientID'], ENT_QUOTES);
$Diagnosis = htmlentities($_POST['Diagnosis'], ENT_QUOTES);
$Gender = htmlentities($_POST['Gender'], ENT_QUOTES);
$LevelCare = htmlentities($_POST['LevelCare'], ENT_QUOTES);
$Counselor = htmlentities($_POST['Counselor'], ENT_QUOTES);
// check that FirstName and LastName are both not empty
if ($FirstName == '' || $MiddleName == '' || $LastName == '' || $ClientID == '')
{
// if they are empty, show an error message and display the form
$error = 'ERROR: Please fill in all required fields!';
renderForm($FirstName, $MiddleName, $LastName, $ClientID, $Diagnosis, $Gender, $LevelCare, $Counselor, $error, $id);
}
else
{
// if everything is fine, update the record in the database
if ($stmt = $mysqli->prepare("UPDATE clients SET FirstName = ?, MiddleName = ?, LastName = ?, ClientID = ?, Diagnosis = ?, Gender = ?, LevelCare = ?, Counselor = ?
WHERE id=?"))
{
$stmt->bind_param("sssisss", $FirstName, $MiddleName, $LastName, $ClientID, $Diagnosis, $Gender, $LevelCare, $counselor, $id);
$stmt->execute();
$stmt->close();
}
// show an error message if the query has an error
else
{
echo "ERROR: could not prepare SQL statement.";
}
// redirect the user once the form is updated
header("Location: view.php");
}
}
// if the 'id' variable is not valid, show an error message
else
{
echo "Error!";
}
}
// if the form hasn't been submitted yet, get the info from the database and show the form
else
{
// make sure the 'id' value is valid
if (is_numeric($_GET['id']) && $_GET['id'] > 0)
{
// get 'id' from URL
$id = $_GET['id'];
// get the recod from the database
if($stmt = $mysqli->prepare("SELECT * FROM clients WHERE id=?"))
{
$stmt->bind_param("i", $id);
$stmt->execute();
$stmt->bind_result($id, $FirstName, $MiddleName, $LastName, $ClientID, $Diagnosis, $Gender, $LevelCare, $Counselor);
$stmt->fetch();
// show the form
renderForm($FirstName, $MiddleName, $LastName, $ClientID, $Diagnosis, $Gender, $LevelCare, $Counselor, NULL, $id);
$stmt->close();
}
// show an error if the query has an error
else
{
echo "Error: could not prepare SQL statement";
}
}
// if the 'id' value is not valid, redirect the user back to the view.php page
else
{
header("Location: view.php");
}
}
}
/*
NEW RECORD
*/
// if the 'id' variable is not set in the URL, we must be creating a new record
else
{
// if the form's submit button is clicked, we need to process the form
if (isset($_POST['submit']))
{
// get the form data
$FirstName = htmlentities($_POST['FirstName'], ENT_QUOTES);
$MiddleName = htmlentities($_POST['MiddleName'], ENT_QUOTES);
$LastName = htmlentities($_POST['LastName'], ENT_QUOTES);
$ClientID = htmlentities($_POST['ClientID'], ENT_QUOTES);
$Diagnosis = htmlentities($_POST['Diagnosis'], ENT_QUOTES);
$Gender = htmlentities($_POST['Gender'], ENT_QUOTES);
$LevelCare = htmlentities($_POST['LevelCare'], ENT_QUOTES);
$Counselor = htmlentities($_POST['Counselor'], ENT_QUOTES);
// check that FirstName and LastName are both not empty
if ($FirstName == '' || $MiddleName == '' || $LastName == '' || $ClientID == '')
{
// if they are empty, show an error message and display the form
$error = 'ERROR: Please fill in all required fields!';
renderForm($FirstName, $MiddleName, $LastName, $ClientID, $Diagnosis, $Gender, $LevelCare, $LevelCare, $Counselor, $error);
}
else
{
// insert the new record into the database
if ($stmt = $mysqli->prepare("INSERT clients (FirstName, MiddleName, LastName, ClientID, Diagnosis, Gender, LevelCare, Counselor) VALUES (?, ?, ?, ?, ?, ?, ?, ?)"))
{
$stmt->bind_param("ssssssss", $FirstName, $MiddleName, $LastName, $ClientID, $Diagnosis, $Gender, $LevelCare, $Counselor);
$stmt->execute();
$stmt->close();
}
// show an error if the query has an error
else
{
echo "ERROR: Could not prepare SQL statement.";
}
// redirec the user
header("Location: view.php");
}
}
// if the form hasn't been submitted yet, show the form
else
{
renderForm();
}
}
// close the mysqli connection
$mysqli->close();
?>
How Do I Style This?
in PHP
Posted
How do I style this table to make it look "prettier" I have tried a little with css and html tags with no luck.